short int 转bit
时间: 2023-10-24 17:12:26 浏览: 39
可以通过位运算将 short int 转换为 bit。具体方法是用左移运算符将 short int 每一位依次左移,并用位与运算符将其与 1 进行比较,得到相应的 bit 值。例如,下面的代码将 short int 转换为 16 位的 bit 序列:
```
#include <stdio.h>
void int2bit(short num) {
for (int i = 15; i >= 0; i--) {
printf("%d", (num >> i) & 1);
}
printf("\n");
}
int main() {
short num = 0xE3A1;
int2bit(num);
return 0;
}
```
输出结果为:
```
1110001110100001
```
相关问题
unsigned short int
An unsigned short int is a data type that can hold a range of positive integers from 0 to 65,535. It is a 16-bit data type, which means it occupies 2 bytes of memory. The "unsigned" keyword indicates that it can only hold non-negative values and does not allow negative numbers to be stored in it. It is commonly used in programs where memory space is a concern and positive values are expected.
linux下c++short转二进制
在Linux下,我们可以使用C语言编写程序来将short类型的数据转换为二进制形式。下面是一个简单的例子:
```c
#include <stdio.h>
// 函数将给定的short类型数据转换为二进制
void shortToBinary(short num) {
int binary[16]; // 用于存储二进制的数组
// 将二进制数组初始化为0
for (int i = 0; i < 16; i++) {
binary[i] = 0;
}
int index = 0;
while (num > 0) {
binary[index++] = num % 2;
num = num / 2;
}
// 反向打印二进制数组
for (int i = 15; i >= 0; i--) {
printf("%d", binary[i]);
}
printf("\n");
}
int main() {
short num = 10; // 待转换的short类型数据
shortToBinary(num);
return 0;
}
```
在这个例子中,我们定义了一个函数`shortToBinary`,它将给定的short类型数据转换为对应的二进制形式并打印出来。我们在主函数中调用这个函数,传入待转换的short类型数据。运行程序,将会输出10的二进制形式:0000000000001010。这是因为short类型数据在内存中占用16个bit,所以输出了16位的二进制数。
这只是一个简单的例子,你可以根据自己的实际需求来修改程序。
相关推荐
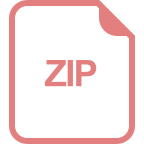
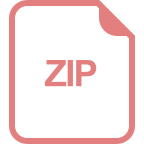
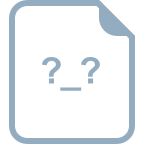












