本题要求实现一个函数,遍历链表求链表节点数据的累加和 节点类型定义: struct node { int ch ; struct node *next ;}
时间: 2024-03-06 21:52:02 浏览: 31
好的,下面是遍历链表求节点数据累加和的函数实现:
```c
int sumOfNodes(struct node* head) {
int sum = 0;
struct node* curr = head;
while (curr != NULL) {
sum += curr->ch;
curr = curr->next;
}
return sum;
}
```
这个函数会从链表头节点开始遍历整个链表,将节点数据累加到变量 `sum` 中,最后返回累加和。需要注意的是,函数中的 `struct node` 是链表节点的数据类型定义,`head` 是链表头节点的指针。
相关问题
本题要求实现一个函数,遍历链表求链表节点数据的最大值 节点类型定义: struct node { int ch ; struct node *next ;}
可以按照以下步骤来实现遍历链表求链表节点数据的最大值:
1. 定义一个变量maxVal,初始值为链表的头节点的值。
2. 遍历链表,比较每个节点的值和maxVal,如果当前节点的值大于maxVal,则将maxVal赋值为当前节点的值。
3. 遍历完成后,maxVal的值就是链表中的最大值。
下面是代码实现:
```c
struct node {
int ch;
struct node* next;
};
int max_node(struct node* head) {
if (head == NULL) {
return -1;
}
int maxVal = head->ch;
struct node* curNode = head->next;
while (curNode != NULL) {
if (curNode->ch > maxVal) {
maxVal = curNode->ch;
}
curNode = curNode->next;
}
return maxVal;
}
```
使用时,只需要调用max_node函数并传入链表的头节点即可:
```c
struct node* head = createList(); // 创建链表
int maxVal = max_node(head); // 查找最大值
printf("Max value: %d\n", maxVal);
```
本题要求实现一个函数,遍历一个不带头结点的链表,求链表节点数据的累加和 节点类型定义: struct node { int ch ; struct node *next
可以使用一个指针变量遍历链表,同时维护一个累加和变量,每次遍历到的节点数据加上到累加和中。以下是一个可能的实现:
```c
int findSumNode(struct node *head) {
struct node *p = head;
int sum = 0;
while (p != NULL) {
sum += p->ch;
p = p->next;
}
return sum;
}
```
在这个函数中,我们首先将指针变量p指向链表的第一个节点,并将累加和变量sum初始化为0。然后,我们使用一个while循环遍历链表,每次遍历到一个节点,就将该节点的数据加到累加和中。最后,我们返回累加和即可。需要注意的是,这个函数假设链表至少包含一个节点,否则会出现错误。
相关推荐
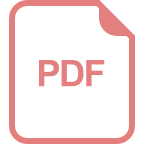
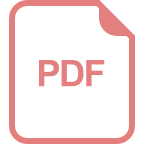
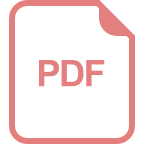











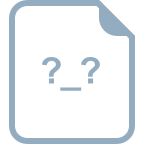
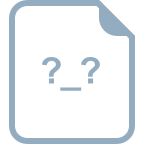