lin协议 主机任务状态机verilog代码
时间: 2023-07-23 15:07:17 浏览: 393
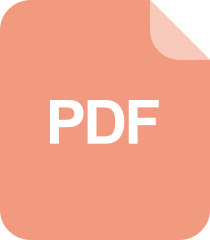
Verilog代码描述对状态机综合的研究
以下是一个简单的基于 Lin 2.0 协议的主机任务状态机的 Verilog 代码示例:
```
module lin_master_task_state_machine(
input clk,
input reset,
input tx_en,
input rx_en,
input tx_data,
output reg tx_ready,
output reg tx_break,
output reg[7:0] rx_data,
output reg rx_complete,
output reg rx_error
);
// LIN 2.0 protocol states
typedef enum {
IDLE,
BREAK,
SYNCH,
HEADER,
DATA,
CHECKSUM
} lin_state_t;
// Task state machine states
typedef enum {
INIT,
TX,
RX
} task_state_t;
// Variables to hold task state machine state and LIN protocol state
reg[1:0] task_state;
reg[2:0] lin_state;
// Variables to hold header and data bytes
reg[5:0] header;
reg[7:0] data[8];
// Variables to hold checksum and checksum validation
reg[7:0] checksum;
reg[1:0] checksum_state;
// Constants for break and sync intervals
parameter BREAK_TIME = 104;
parameter SYNC_TIME = 13;
// Counter for break and sync intervals
reg[6:0] counter;
// Initialize task state machine and LIN protocol state
initial begin
task_state = INIT;
lin_state = IDLE;
end
// Main state machine
always @(posedge clk) begin
if (reset) begin
task_state <= INIT;
lin_state <= IDLE;
tx_ready <= 1'b0;
tx_break <= 1'b0;
rx_data <= 8'd0;
rx_complete <= 1'b0;
rx_error <= 1'b0;
counter <= 7'd0;
end
else begin
case (task_state)
INIT:
if (tx_en) begin
task_state <= TX;
lin_state <= BREAK;
tx_ready <= 1'b1;
tx_break <= 1'b1;
counter <= BREAK_TIME;
end
else if (rx_en) begin
task_state <= RX;
lin_state <= IDLE;
end
TX:
case (lin_state)
BREAK:
if (counter == 7'd0) begin
lin_state <= SYNCH;
tx_break <= 1'b0;
counter <= SYNC_TIME;
end
else begin
counter <= counter - 1'd1;
end
SYNCH:
if (counter == 7'd0) begin
lin_state <= HEADER;
header <= 6'b100000;
counter <= 7'd5;
end
else begin
counter <= counter - 1'd1;
end
HEADER:
if (counter == 7'd0) begin
lin_state <= DATA;
data[0] <= tx_data;
counter <= 7'd8;
end
else begin
counter <= counter - 1'd1;
end
DATA:
if (counter == 7'd0) begin
if (checksum_state == 2'd0) begin
lin_state <= CHECKSUM;
checksum <= header + tx_data;
for (int i = 0; i < 8; i = i + 1) begin
checksum <= checksum + data[i];
end
checksum_state <= 2'd1;
counter <= 7'd8;
end
else begin
lin_state <= IDLE;
tx_ready <= 1'b0;
task_state <= INIT;
end
end
else begin
data[counter-1] <= tx_data;
counter <= counter - 1'd1;
end
CHECKSUM:
if (counter == 7'd0) begin
if (checksum_state == 2'd1) begin
lin_state <= IDLE;
tx_ready <= 1'b0;
task_state <= INIT;
end
else begin
rx_complete <= 1'b1;
counter <= 7'd8;
end
end
else begin
checksum <= checksum + rx_data;
counter <= counter - 1'd1;
end
endcase
RX:
case (lin_state)
IDLE:
if (rx_en) begin
lin_state <= BREAK;
rx_complete <= 1'b0;
rx_error <= 1'b0;
counter <= BREAK_TIME;
end
BREAK:
if (rx_en) begin
if (counter == 7'd0) begin
lin_state <= SYNCH;
counter <= SYNC_TIME;
end
else begin
counter <= counter - 1'd1;
end
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
SYNCH:
if (rx_en) begin
if (counter == 7'd0) begin
lin_state <= HEADER;
header <= rx_data;
checksum <= header;
checksum_state <= 2'd1;
counter <= 7'd5;
end
else begin
counter <= counter - 1'd1;
end
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
HEADER:
if (rx_en) begin
if (counter == 7'd0) begin
lin_state <= DATA;
data[0] <= rx_data;
checksum <= checksum + rx_data;
counter <= 7'd8;
end
else begin
counter <= counter - 1'd1;
end
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
DATA:
if (rx_en) begin
if (counter == 7'd0) begin
if (checksum_state == 2'd1) begin
lin_state <= CHECKSUM;
checksum <= checksum + rx_data;
checksum_state <= 2'd2;
counter <= 7'd8;
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
end
else begin
data[counter-1] <= rx_data;
checksum <= checksum + rx_data;
counter <= counter - 1'd1;
end
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
CHECKSUM:
if (rx_en) begin
if (counter == 7'd0) begin
if (checksum_state == 2'd2 && checksum == 8'd0) begin
rx_complete <= 1'b1;
lin_state <= IDLE;
task_state <= INIT;
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
end
else begin
checksum <= checksum + rx_data;
counter <= counter - 1'd1;
end
end
else begin
lin_state <= IDLE;
rx_error <= 1'b1;
task_state <= INIT;
end
endcase
endcase
end
end
endmodule
```
请注意,此代码是一个简单示例,并且可能需要根据您的应用程序进行修改和调整。此外,此代码只实现了协议的发送和接收部分,您还需要添加适当的硬件接口和控制逻辑以与您的特定系统集成。
阅读全文
相关推荐
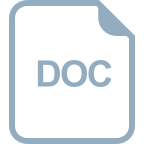
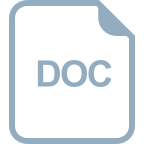
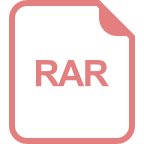
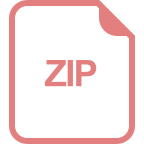
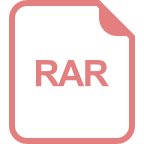
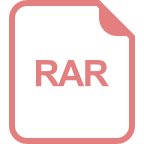
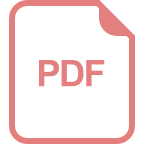
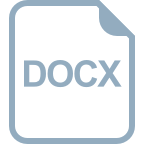
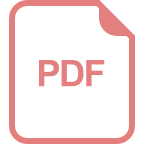


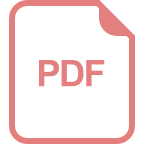
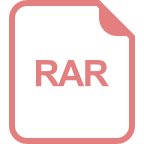
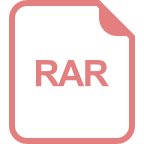
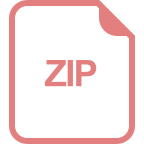
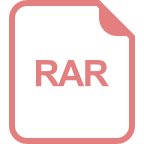