std::string contents((std::istreambuf_iterator<char>(in)), std::istreambuf_iterator<char>());
时间: 2024-05-31 19:13:28 浏览: 11
这段代码是用来读取一个文件的全部内容并存储到一个字符串中。
具体实现是通过一个 std::istreambuf_iterator 对象,将文件输入流 in 的内容逐个字符读入,并通过 std::string 构造函数将其存储到字符串 contents 中。
这里使用了两个 std::istreambuf_iterator<char> 对象,第一个作为 std::string 构造函数的起始位置,第二个作为输入流结束位置。当读取到输入流末尾时,输入流迭代器会等同于 std::istreambuf_iterator<char>(),此时构造函数会自动结束字符串的构造。
需要注意的是,这段代码仅适用于文本文件。对于二进制文件的读取,应当使用另外的方法。
相关问题
解释string content((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
这行代码是将一个文件的内容读取到一个字符串变量中。具体来说,它的作用是:
1. 创建一个std::istreambuf_iterator<char>类型的对象,该对象用于从文件流中读取字符。
2. 将该对象作为std::string构造函数的第一个参数,表示从该对象指向的位置开始读取字符。
3. 创建另一个std::istreambuf_iterator<char>类型的对象,该对象表示文件流的结尾位置。
4. 将该对象作为std::string构造函数的第二个参数,表示读取字符的结束位置。
因此,这行代码的作用相当于从文件流中读取所有字符,并将它们存储到一个字符串变量content中。
需要注意的是,该代码使用了C++标准库中的迭代器和构造函数,可以方便地将文件内容读取到一个字符串变量中。此外,该代码可以用于读取任意类型的文件,包括文本文件和二进制文件。
上述代码使用std::string content((std::istreambuf_iterator<char>(ifs)), (std::istreambuf_iterator<char>()));
是的,您可以使用 `std::istreambuf_iterator` 一次性获取整个文件的内容,并将其存储在 `std::string` 中。这种方法使用了 C++ 中的 STL(标准模板库)。
以下是使用 `std::istreambuf_iterator` 的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
string filename = "example.txt";
string content = "";
ifstream input(filename);
if (input) {
content.assign((istreambuf_iterator<char>(input)), (istreambuf_iterator<char>()));
}
input.close();
cout << "File content:\n" << content << endl;
return 0;
}
```
在这个示例中,我们首先定义了文件名和一个字符串来存储文件内容。我们打开文件并检查文件是否成功打开。如果文件已成功打开,则使用 `std::istreambuf_iterator` 一次性获取整个文件的内容,并将其存储在内容字符串中。最后,我们关闭文件并输出文件内容。
请注意,在使用 `std::istreambuf_iterator` 时,我们使用了 `std::string` 的 `assign` 函数来将文件内容分配给内容字符串。 `assign` 函数将迭代器的起始和结束位置作为参数。
相关推荐
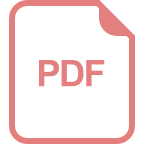
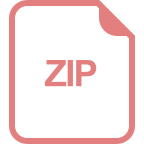
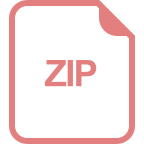












