application.properties配置mysql
时间: 2023-05-31 22:21:06 浏览: 170
### 回答1:
application.properties是Spring Boot项目中的配置文件,用于配置应用程序的各种属性。下面是配置MySQL数据库的示例:
# 数据库配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=false
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# JPA配置
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect
其中,spring.datasource.url是数据库连接字符串,spring.datasource.username和spring.datasource.password是数据库的用户名和密码,spring.datasource.driver-class-name是数据库驱动程序的类名。spring.jpa.show-sql用于显示SQL语句,spring.jpa.hibernate.ddl-auto用于自动创建表,spring.jpa.properties.hibernate.dialect用于指定Hibernate方言。
### 回答2:
在项目开发中,我们经常需要使用到数据库来保存数据,而MySQL作为一个常用的关系型数据库系统,使用非常广泛。在Spring Boot项目中,我们可以通过在application.properties文件中配置MySQL来方便地进行数据库操作。
首先,我们需要引入MySQL驱动包,可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
```
接下来,可以在application.properties文件中进行MySQL的配置,具体步骤如下:
1. 配置数据源相关信息,包括数据库地址、端口、用户名、密码等:
```java
spring.datasource.url=jdbc:mysql://localhost:3306/test_db?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
- spring.datasource.url:数据库连接地址,其中test_db是数据库名;
- spring.datasource.username:连接MySQL的用户名;
- spring.datasource.password:连接MySQL的密码;
- spring.datasource.driver-class-name:指定连接的数据库驱动。
2. 配置JPA相关信息:
```java
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect
```
- spring.jpa.show-sql:可以将数据的SQL语句输出到控制台方便调试;
- spring.jpa.hibernate.ddl-auto:指定启动时是否自动创建表结构,update表示有更改时自动更新;
- spring.jpa.properties.hibernate.dialect:指定使用的数据库方言,这里使用的是MySQL5。
至此,我们已经完成了MySQL相关的配置工作。在代码中,可以通过注入DataSource或JdbcTemplate来进行数据库操作。例如:
```java
@Autowired
private JdbcTemplate jdbcTemplate;
public List<User> getAllUsers() {
String sql = "SELECT * FROM user";
List<User> users = jdbcTemplate.query(sql, new UserMapper());
return users;
}
```
需要注意的是,以上仅为MySQL的最基本配置,实际应用中还需要考虑数据源的连接池和事务等方面的配置,以确保项目的性能和数据完整性。
### 回答3:
application.properties是Spring Boot项目的配置文件,用于管理项目的配置信息。其中,关于mysql的配置可以分为以下几个方面:
1. 数据库连接信息:
spring.datasource.url=jdbc:mysql://localhost:3306/test
spring.datasource.username=root
spring.datasource.password=123456
其中,spring.datasource.url表示数据库的连接地址,3306是mysql数据库的默认端口,test是数据库的名称;spring.datasource.username和spring.datasource.password分别表示数据库的账号和密码。
2. 数据库连接池:
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.initialSize=5
spring.datasource.maxActive=10
spring.datasource.maxWait=10000
其中,spring.datasource.driver-class-name是mysql的驱动名;spring.datasource.initialSize表示初始化连接池时建立的连接数;spring.datasource.maxActive表示连接池中可用的最大连接数;spring.datasource.maxWait表示获取连接时最长的等待时间,单位为毫秒。
3. 数据库方言:
spring.jpa.database-platform=org.hibernate.dialect.MySQL5Dialect
这里指定了使用mysql5.x的方言,这样可以确保使用mysql数据库时,Hibernate可以正确地生成SQL语句。
4. 数据库表的自动创建和更新:
spring.jpa.hibernate.ddl-auto=create
这里的spring.jpa.hibernate.ddl-auto属性表示Hibernate在启动时根据实体类自动创建表,也可以设置为update表示等到实体类变化时再更新表结构。
综上所述,可以看出,在application.properties中配置mysql需要关注的方面较多,需要根据实际项目的需求进行相应的配置。配置时应该注意账号密码的安全性,避免在代码库中出现硬编码的敏感信息,可以使用环境变量或配置中心等方式进行管理。同时,应该通过连接池等手段为程序提供可靠的数据库连接,提高应用的性能和稳定性。
阅读全文
相关推荐



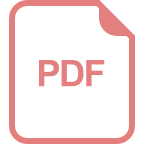











