用python编写一个双阈值确定图像边缘的代码,使用递归的方法,不使用opencv库
时间: 2024-05-08 19:17:22 浏览: 61
以下是使用Python递归方法实现双阈值确定图像边缘的代码:
```python
import numpy as np
from PIL import Image
def double_threshold(image, low_threshold, high_threshold):
# 获取图像大小
height, width = image.shape
# 定义输出图像
output_image = np.zeros((height, width), dtype=np.uint8)
# 递归函数,用于搜索边缘
def search_edge(x, y):
# 检查像素是否已经处理过
if output_image[x][y] != 0:
return
# 将当前像素标记为处理过
output_image[x][y] = 255
# 检查当前像素是否在高阈值范围内
if image[x][y] >= high_threshold:
# 搜索当前像素周围的像素
for i in range(-1, 2):
for j in range(-1, 2):
# 检查像素是否在图像范围内
if x+i < 0 or y+j < 0 or x+i >= height or y+j >= width:
continue
# 递归搜索周围像素
search_edge(x+i, y+j)
# 检查当前像素是否在低阈值范围内
elif image[x][y] >= low_threshold:
# 搜索当前像素周围的像素
for i in range(-1, 2):
for j in range(-1, 2):
# 检查像素是否在图像范围内
if x+i < 0 or y+j < 0 or x+i >= height or y+j >= width:
continue
# 递归搜索周围像素
search_edge(x+i, y+j)
# 遍历图像像素
for i in range(height):
for j in range(width):
# 检查像素是否已经处理过
if output_image[i][j] != 0:
continue
# 递归搜索边缘
search_edge(i, j)
return output_image
# 加载图像
image = np.array(Image.open('image.png').convert('L'))
# 双阈值确定边缘
edge_image = double_threshold(image, 50, 100)
# 显示图像
Image.fromarray(edge_image).show()
```
在这个代码中,我们定义了一个名为`double_threshold`的函数,它接收三个参数:图像、低阈值和高阈值。该函数返回一个包含图像边缘的二值图像。
在该函数中,我们定义了一个名为`search_edge`的递归函数,用于搜索边缘。该函数接收两个参数:当前像素的坐标x和y。首先,该函数检查当前像素是否已经处理过,如果已经处理过,则直接返回。然后,该函数将当前像素标记为处理过的像素,并检查当前像素是否在高阈值范围内。如果是,则搜索当前像素周围的像素,并递归搜索周围的像素。如果当前像素在低阈值范围内,则搜索当前像素周围的像素,并递归搜索周围的像素。
在`double_threshold`函数中,我们遍历图像像素,并检查像素是否已经处理过。如果像素已经处理过,则跳过该像素。否则,我们调用`search_edge`函数来搜索边缘。
最后,我们将返回的二值图像保存为PNG格式,并使用PIL库的`Image`类将其显示出来。
阅读全文
相关推荐



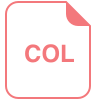
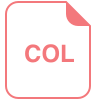
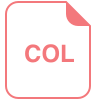
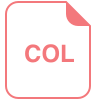


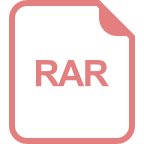
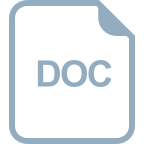
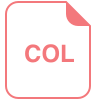
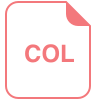
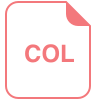
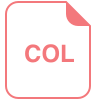
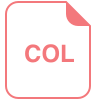