bool YuFa(const vector<pair<string, string>>& tokens) { stack<string> parenthesesStack; int position = 0; for (auto token : tokens) { position++; if (token.first == "Parenthesis") { if (token.second == "(") { parenthesesStack.push("("); } else { // ")" if (parenthesesStack.empty() || parenthesesStack.top() != "(") { cout << "括号不匹配,出错位置:" << position << endl; return false; // 括号不匹配 } parenthesesStack.pop(); } } } if (!parenthesesStack.empty()) { cout << "括号不匹配,出错位置:" << position << endl; return false; }else{ cout<<"括号匹配成功"<< endl; return true; } } // 词法分析器 vector<pair<string, string>> lexer(string expression) { vector<pair<string, string>> tokens; string token = ""; for (char c : expression) { if (isOperator(c)) { if (!token.empty()) { tokens.push_back(make_pair("Operand", token)); token = ""; } tokens.push_back(make_pair("Operator", string(1, c))); } else if (Bracket(c)) { if (!token.empty()) { tokens.push_back(make_pair("Operand", token)); token = ""; } tokens.push_back(make_pair("Parenthesis", string(1, c))); } else if (c != ' ') { token += c; } } if (!token.empty()) { tokens.push_back(make_pair("Operand", token)); } return tokens; }分析这段代码的数据类型定义和存储结构
时间: 2024-02-14 18:20:18 浏览: 23
该段代码中涉及到的数据类型定义和存储结构包括:
1. vector<pair<string, string>>:一个存储了一组键-值对的动态数组,其中每个键-值对都由两个字符串组成,分别表示词法分析器分析出的词法单元类型和对应的字符串值。
2. stack<string>:一个栈,用于存储左括号"(",并在遇到右括号")"时进行出栈操作以判断括号是否匹配。
3. string:一个字符串类型,用于临时存储词法分析器分析出的操作数或运算符。
4. char:一个字符类型,用于遍历表达式字符串并逐个判断字符类型。
相关问题
auto cmp = [](const pair<int, int>& a, const pair<int, int>& b) -> bool { return a.second < b.second; }; priority_queue<pair<int, int>, vector<pair<int, int>>, decltype(cmp)> que(cmp);
这段代码是用来定义一个带有自定义比较器的优先队列priority_queue的,其中:
- auto cmp = [](const pair<int, int>& a, const pair<int, int>& b) -> bool { return a.second < b.second; }; 定义了一个lambda表达式cmp,用来比较两个pair<int, int>类型的元素,按照第二个元素从小到大排序。
- decltype(cmp)是用于指定priority_queue的第三个参数,即比较器类型,这里为decltype(cmp),也就是cmp的类型。
- vector<pair<int, int>>表示priority_queue内部使用的容器类型,即存储元素的容器类型。
- priority_queue<pair<int, int>, vector<pair<int, int>>, decltype(cmp)> que(cmp); 定义了一个名为que的priority_queue对象,其元素类型为pair<int, int>,容器类型为vector<pair<int, int>>,比较器类型为cmp。这个对象在创建时使用了cmp作为比较器,所以插入元素时会按照cmp定义的比较规则进行排序。
怎么对unordered_map<int,vector<string>> 类型排序,排序顺序根据 unordered_map<int,vector<string>>类型里的int来定
对于unordered_map<int,vector<string>>类型的排序,可以使用std::sort函数结合自定义的比较函数来实现。比较函数需要按照unordered_map<int,vector<string>>中的int进行排序,具体实现如下:
```cpp
bool cmp(const pair<int, vector<string>>& a, const pair<int, vector<string>>& b) {
return a.first < b.first;
}
unordered_map<int, vector<string>> myMap;
// 对 myMap 进行排序
vector<pair<int, vector<string>>> sortedVec(myMap.begin(), myMap.end());
sort(sortedVec.begin(), sortedVec.end(), cmp);
```
在以上代码中,我们定义了一个cmp函数来比较unordered_map<int,vector<string>>类型中的int。然后使用std::sort函数对vector<pair<int, vector<string>>>类型进行排序,最终得到的sortedVec就是按照int排序后的结果。
相关推荐
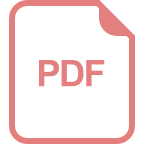
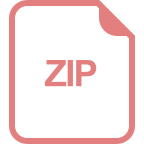














