Java编写五子棋游戏代码
时间: 2023-05-11 16:07:08 浏览: 122
非常感谢您的提问,以下是 Java 编写五子棋游戏代码的示例:
```java
import java.util.Scanner;
public class Gobang {
private static final int BOARD_SIZE = 15;
private String[][] board = new String[BOARD_SIZE][BOARD_SIZE];
private String player1;
private String player2;
public Gobang(String player1, String player2) {
this.player1 = player1;
this.player2 = player2;
initBoard();
}
private void initBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = "+";
}
}
}
public void start() {
Scanner scanner = new Scanner(System.in);
String currentPlayer = player1;
while (true) {
printBoard();
System.out.println(currentPlayer + ",请输入您要下的位置,格式为x,y:");
String input = scanner.nextLine();
String[] positions = input.split(",");
int x = Integer.parseInt(positions[0]);
int y = Integer.parseInt(positions[1]);
if (board[x][y].equals("+")) {
board[x][y] = currentPlayer.equals(player1) ? "O" : "X";
if (isWin(x, y)) {
printBoard();
System.out.println(currentPlayer + "获胜!");
break;
}
currentPlayer = currentPlayer.equals(player1) ? player2 : player1;
} else {
System.out.println("该位置已经有棋子,请重新输入!");
}
}
}
private boolean isWin(int x, int y) {
String symbol = board[x][y];
int count = 1;
int i, j;
// 横向判断
i = x;
j = y - 1;
while (j >= 0 && board[i][j].equals(symbol)) {
count++;
j--;
}
j = y + 1;
while (j < BOARD_SIZE && board[i][j].equals(symbol)) {
count++;
j++;
}
if (count >= 5) {
return true;
}
// 竖向判断
count = 1;
i = x - 1;
j = y;
while (i >= 0 && board[i][j].equals(symbol)) {
count++;
i--;
}
i = x + 1;
while (i < BOARD_SIZE && board[i][j].equals(symbol)) {
count++;
i++;
}
if (count >= 5) {
return true;
}
// 左上到右下判断
count = 1;
i = x - 1;
j = y - 1;
while (i >= 0 && j >= 0 && board[i][j].equals(symbol)) {
count++;
i--;
j--;
}
i = x + 1;
j = y + 1;
while (i < BOARD_SIZE && j < BOARD_SIZE && board[i][j].equals(symbol)) {
count++;
i++;
j++;
}
if (count >= 5) {
return true;
}
// 右上到左下判断
count = 1;
i = x - 1;
j = y + 1;
while (i >= 0 && j < BOARD_SIZE && board[i][j].equals(symbol)) {
count++;
i--;
j++;
}
i = x + 1;
j = y - 1;
while (i < BOARD_SIZE && j >= 0 && board[i][j].equals(symbol)) {
count++;
i++;
j--;
}
if (count >= 5) {
return true;
}
return false;
}
private void printBoard() {
System.out.print(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < BOARD_SIZE; i++) {
System.out.print(i + " ");
for (int j = 0; j < BOARD_SIZE; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
}
```
如果您有任何问题或需要进一步的帮助,请随时联系我。
阅读全文
相关推荐
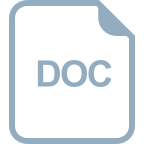
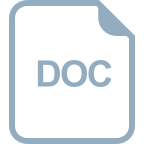
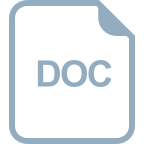
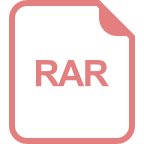
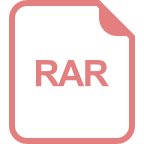

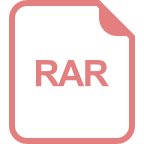
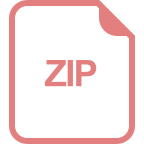
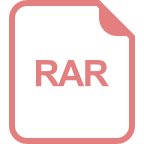
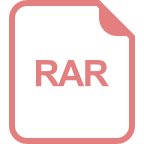
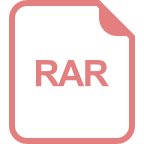
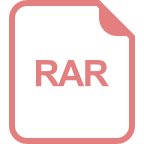
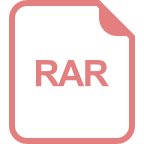
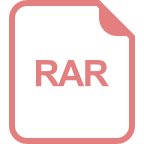

