使用java编写一个五子棋游戏代码
时间: 2023-06-02 07:06:10 浏览: 137
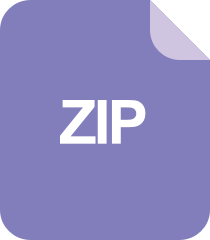
使用java编写的五子棋游戏
好的,关于编写五子棋游戏的代码,我很乐意为您提供帮助和建议。首先,您可以使用Java语言、JavaFX图形界面和面向对象设计的思路,来创建一个简单易用的五子棋游戏程序。以下是一个示例代码的框架:
```
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class Gomoku extends Application {
private final int BOARD_SIZE = 15;
private final int CELL_SIZE = 40;
private int[][] board = new int[BOARD_SIZE][BOARD_SIZE];
public void start(Stage primaryStage) {
GridPane grid = new GridPane();
grid.setPadding(new Insets(10, 10, 10, 10));
grid.setVgap(1);
grid.setHgap(1);
// Create cells for the board
for (int row = 0; row < BOARD_SIZE; row++) {
for (int col = 0; col < BOARD_SIZE; col++) {
Cell cell = new Cell(row, col, CELL_SIZE);
grid.add(cell, col, row);
}
}
Scene scene = new Scene(grid);
primaryStage.setTitle("Gomoku");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
/**
* Inner class representing a single cell in the game board
*/
private class Cell extends Circle {
private final int row;
private final int col;
public Cell(int row, int col, int size) {
super(size / 2);
this.row = row;
this.col = col;
setStroke(Color.BLACK);
setFill(Color.WHITE);
setOnMouseClicked(e -> handleMouseClick());
}
/**
* Handle a mouse click on this cell
*/
private void handleMouseClick() {
if (board[row][col] != 0) {
System.out.println("This cell is already occupied!");
return;
}
board[row][col] = 1; // 1 represents a black stone
// TODO: Place black stone on this cell
// and check if black has won the game
// TODO: Check if game is a draw
// Let the AI place a white stone on the board
// and check if white has won the game
}
}
}
```
这段代码主要是创建了一个JavaFX的界面,并定义了一个内部类Cell,用于表示游戏棋盘上的单元格。在鼠标点击事件处理函数handleMouseClick()中,您需要根据点击的单元格位置,在棋盘上放置黑色的棋子,并判断游戏是否胜利或平局。而与之配合的程序AI同样可以在handleMouseClick()事件处理函数内部加入。总体而言,本段代码只是一个简单的示例,您还需要进一步完善细节部分。希望对您有所帮助!
阅读全文
相关推荐
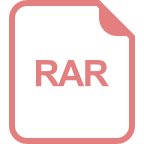

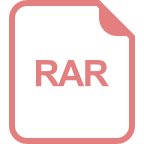
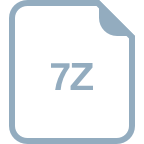









