用java编写一个五子棋游戏
时间: 2023-08-24 17:09:44 浏览: 39
抱歉,作为AI语言模型,我无法编写可执行的Java程序。但我可以提供一个五子棋游戏的代码框架,供您参考和学习。
```
import java.util.Scanner;
public class Gomoku {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int[][] board = new int[15][15]; // 棋盘
boolean isBlack = true; // 当前是否是黑子下
boolean isOver = false; // 游戏是否结束
while (!isOver) {
// 打印棋盘
printBoard(board);
// 提示当前哪方下子
System.out.println(isBlack ? "黑方下子" : "白方下子");
// 接收用户输入
int x = scanner.nextInt();
int y = scanner.nextInt();
// 判断输入是否合法
if (x < 0 || x >= 15 || y < 0 || y >= 15) {
System.out.println("输入不合法,请重新输入");
continue;
}
if (board[x][y] != 0) {
System.out.println("该位置已经有棋子,请重新输入");
continue;
}
// 下子
board[x][y] = isBlack ? 1 : 2;
// 判断是否获胜
if (checkWin(board, x, y)) {
printBoard(board);
System.out.println(isBlack ? "黑方胜利" : "白方胜利");
isOver = true;
}
// 判断是否平局
if (!isOver && isTie(board)) {
printBoard(board);
System.out.println("平局");
isOver = true;
}
// 切换下子方
isBlack = !isBlack;
}
}
// 打印棋盘
public static void printBoard(int[][] board) {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[0].length; j++) {
System.out.print(board[i][j] == 0 ? "┼ " : (board[i][j] == 1 ? "● " : "○ "));
}
System.out.println();
}
System.out.println();
}
// 判断是否获胜
public static boolean checkWin(int[][] board, int x, int y) {
int color = board[x][y];
int count = 1;
// 判断横向
for (int i = x - 1; i >= 0 && board[i][y] == color; i--) count++;
for (int i = x + 1; i < 15 && board[i][y] == color; i++) count++;
if (count >= 5) return true;
// 判断竖向
count = 1;
for (int j = y - 1; j >= 0 && board[x][j] == color; j--) count++;
for (int j = y + 1; j < 15 && board[x][j] == color; j++) count++;
if (count >= 5) return true;
// 判断左上-右下方向
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == color; i--, j--) count++;
for (int i = x + 1, j = y + 1; i < 15 && j < 15 && board[i][j] == color; i++, j++) count++;
if (count >= 5) return true;
// 判断左下-右上方向
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < 15 && board[i][j] == color; i--, j++) count++;
for (int i = x + 1, j = y - 1; i < 15 && j >= 0 && board[i][j] == color; i++, j--) count++;
if (count >= 5) return true;
return false;
}
// 判断是否平局
public static boolean isTie(int[][] board) {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
if (board[i][j] == 0) return false;
}
}
return true;
}
}
```
这是一个基本的五子棋游戏代码框架,可以根据需求进行修改和完善。其中,printBoard()方法用于打印棋盘,checkWin()方法用于判断是否获胜,isTie()方法用于判断是否平局。游戏流程大致为:打印棋盘 -> 接收用户输入 -> 下子 -> 判断是否获胜 -> 判断是否平局 -> 切换下子方 -> 重复以上步骤,直到游戏结束。
相关推荐
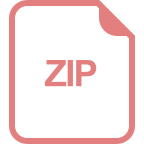
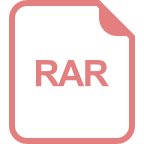
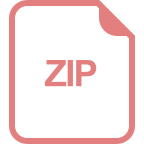














