用Java编写一个五子棋游戏
时间: 2023-05-19 12:04:20 浏览: 90
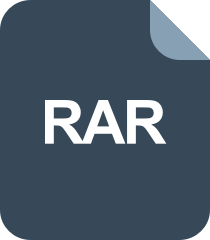
Java编写的五子棋游戏
好的,我可以为您提供一个基本的五子棋游戏的Java代码,如下所示:
```
import java.util.Scanner;
public class GomokuGame {
private static final int BOARD_SIZE = 15;
private static final char EMPTY = '-';
private static final char BLACK = 'X';
private static final char WHITE = 'O';
private char[][] board;
private char currentPlayer;
public GomokuGame() {
board = new char[BOARD_SIZE][BOARD_SIZE];
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = EMPTY;
}
}
currentPlayer = BLACK;
}
public void play() {
Scanner scanner = new Scanner(System.in);
int row, col;
while (true) {
System.out.println("Current player: " + currentPlayer);
printBoard();
System.out.print("Enter row and column (e.g. 3 4): ");
row = scanner.nextInt();
col = scanner.nextInt();
if (isValidMove(row, col)) {
board[row][col] = currentPlayer;
if (isWinningMove(row, col)) {
System.out.println(currentPlayer + " wins!");
printBoard();
break;
}
if (isBoardFull()) {
System.out.println("Game over. It's a tie!");
printBoard();
break;
}
currentPlayer = (currentPlayer == BLACK) ? WHITE : BLACK;
} else {
System.out.println("Invalid move. Try again.");
}
}
}
private boolean isValidMove(int row, int col) {
return (row >= 0 && row < BOARD_SIZE && col >= 0 && col < BOARD_SIZE && board[row][col] == EMPTY);
}
private boolean isWinningMove(int row, int col) {
return (checkRow(row) || checkCol(col) || checkDiagonal(row, col) || checkAntiDiagonal(row, col));
}
private boolean checkRow(int row) {
int count = 0;
for (int i = 0; i < BOARD_SIZE; i++) {
if (board[row][i] == currentPlayer) {
count++;
if (count == 5) {
return true;
}
} else {
count = 0;
}
}
return false;
}
private boolean checkCol(int col) {
int count = 0;
for (int i = 0; i < BOARD_SIZE; i++) {
if (board[i][col] == currentPlayer) {
count++;
if (count == 5) {
return true;
}
} else {
count = 0;
}
}
return false;
}
private boolean checkDiagonal(int row, int col) {
int count = 0;
int i = row, j = col;
while (i >= 0 && j >= 0) {
if (board[i][j] == currentPlayer) {
count++;
if (count == 5) {
return true;
}
} else {
break;
}
i--;
j--;
}
i = row + 1;
j = col + 1;
while (i < BOARD_SIZE && j < BOARD_SIZE) {
if (board[i][j] == currentPlayer) {
count++;
if (count == 5) {
return true;
}
} else {
break;
}
i++;
j++;
}
return false;
}
private boolean checkAntiDiagonal(int row, int col) {
int count = 0;
int i = row, j = col;
while (i >= 0 && j < BOARD_SIZE) {
if (board[i][j] == currentPlayer) {
count++;
if (count == 5) {
return true;
}
} else {
break;
}
i--;
j++;
}
i = row + 1;
j = col - 1;
while (i < BOARD_SIZE && j >= 0) {
if (board[i][j] == currentPlayer) {
count++;
if (count == 5) {
return true;
}
} else {
break;
}
i++;
j--;
}
return false;
}
private boolean isBoardFull() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == EMPTY) {
return false;
}
}
}
return true;
}
private void printBoard() {
System.out.print(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < BOARD_SIZE; i++) {
System.out.print(i + " ");
for (int j = 0; j < BOARD_SIZE; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
GomokuGame game = new GomokuGame();
game.play();
}
}
```
希望这个代码对您有所帮助!
阅读全文
相关推荐
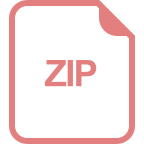









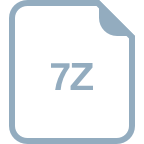
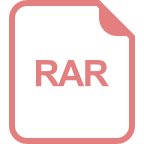
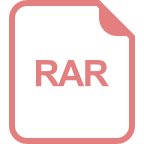
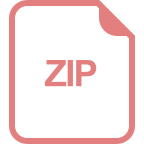


