对下列各行代码进行注释
时间: 2023-07-09 12:36:08 浏览: 85
1.
```
print("Hello, World!")
```
这行代码会打印出 "Hello, World!",是一个简单的输出语句。
2.
```
x = 5
y = 10
print(x + y)
```
这行代码定义了变量 x 和 y,并将它们的值分别设置为 5 和 10。然后,它将 x 和 y 相加,并输出结果 15。
3.
```
for i in range(5):
print(i)
```
这行代码使用 for 循环输出从 0 到 4 的整数。在每次循环中,变量 i 的值会自动更新为下一个整数,并将其打印到屏幕上。
4.
```
def square(x):
return x * x
print(square(4))
```
这行代码定义了一个名为 square 的函数,它接受一个参数 x,并返回 x 的平方。然后,它调用 square 函数并将参数设置为 4,并将结果 16 打印到屏幕上。
5.
```
if x > y:
print("x is greater than y")
else:
print("y is greater than x")
```
这行代码使用 if/else 语句比较 x 和 y 的值。如果 x 大于 y,则打印出 "x is greater than y",否则打印出 "y is greater than x"。
相关问题
给你一个 m x n 的矩阵,矩阵中的数字 各不相同 。请你按 任意 顺序返回矩阵中的所有幸运数。幸运数 是指矩阵中满足同时下列两个条件的元素:在同一行的所有元素中最小,在同一列的所有元素中最大.写出c++代码并附有注释
```cpp
#include <vector>
#include <algorithm> // 用于max_element和min_element函数
class Solution {
public:
std::vector<int> findLucky(std::vector<std::vector<int>>& matrix) {
std::vector<int> lucky_numbers; // 存储幸运数的向量
for (int i = 0; i < matrix.size(); ++i) { // 遍历每一行
int row_min = *std::min_element(matrix[i].begin(), matrix[i].end()); // 找到行内的最小值
for (int j = 0; j < matrix[0].size(); ++j) { // 遍历每一列
if (matrix[j][i] == row_min && matrix[j][i] == *std::max_element(&matrix[j][0], &matrix[j][i + 1])) { // 如果元素既是行内的最小值又是列内的最大值
lucky_numbers.push_back(matrix[j][i]); // 添加到幸运数列表中
}
}
}
return lucky_numbers; // 返回所有找到的幸运数
}
};
// 示例:
// matrix = [[3,7,8],[9,11,13],[15,16,17]]
// 返回:[15]
```
在这个C++代码中,我们首先创建了一个`Solution`类,并在其中定义了一个名为`findLucky`的公共成员函数,它接收一个二维整数矩阵作为输入。然后,我们遍历矩阵的每一行(`for (int i ...)`),找出每行的最小值。接着,对每一列进行内层循环(`for (int j ...)`),检查当前元素是否既是该行的最小值也是该列的最大值。如果是,则将其添加到`lucky_numbers`向量中。最后,函数返回这个包含所有幸运数的向量。
现有某省份21个城市的GDP数据,数据文件储存在文件”gdp_data.txt”中(文件已确保与可执行文件在同一目录下),储存格式如下: 1 ShenZhen 32387.7 1768 2 GuangZhou 28839.0 1881 3 Foshan 12698.4 961 ……依此类推 其中,每行的四项数据分别代表:城市序号、城市名称、城市GDP总量(单位为亿元)、城市常驻人口数(单位为万人)。 在先前的提问中,我们已经使用结构体储存了文件中的城市数据。 现在,请使用C++程序实现下列要求: 1.计算每个城市的人均GDP 2.输出城市人均GDP的最大值和最小值、以及对应城市的名字 注意: 1.上述代码实现需辅以注释解释每行代码的含义与作用 2.上述代码的输出结果均需辅以必要的文字说明 3.输出代码后,请详细解释代码中每一部分的含义、作用和其相关概念。
好的,我会根据你的要求进行回答。下面是实现要求的C++代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义城市结构体
struct City {
int id; // 城市序号
string name; // 城市名称
double gdp; // 城市GDP总量(单位为亿元)
int population; // 城市常驻人口数(单位为万人)
};
int main() {
// 打开数据文件
ifstream infile("gdp_data.txt");
if (!infile) {
cout << "文件打开失败!" << endl;
return 0;
}
// 读取数据文件中的城市数据
City cities[21];
for (int i = 0; i < 21; i++) {
infile >> cities[i].id >> cities[i].name >> cities[i].gdp >> cities[i].population;
}
infile.close();
// 计算每个城市的人均GDP并输出
cout << "各城市人均GDP如下:" << endl;
for (int i = 0; i < 21; i++) {
double perGdp = cities[i].gdp * 10000 / cities[i].population; // 人均GDP计算公式
cout << cities[i].name << ":" << perGdp << "元/人" << endl;
}
// 计算人均GDP的最大值和最小值以及对应城市的名字并输出
double maxPerGdp = cities[0].gdp * 10000 / cities[0].population; // 初始化最大值和最小值
double minPerGdp = cities[0].gdp * 10000 / cities[0].population;
string maxCity = cities[0].name;
string minCity = cities[0].name;
for (int i = 1; i < 21; i++) {
double perGdp = cities[i].gdp * 10000 / cities[i].population; // 人均GDP计算公式
if (perGdp > maxPerGdp) { // 更新最大值和对应城市名字
maxPerGdp = perGdp;
maxCity = cities[i].name;
}
if (perGdp < minPerGdp) { // 更新最小值和对应城市名字
minPerGdp = perGdp;
minCity = cities[i].name;
}
}
cout << "人均GDP最大值为:" << maxPerGdp << "元/人,对应城市为:" << maxCity << endl;
cout << "人均GDP最小值为:" << minPerGdp << "元/人,对应城市为:" << minCity << endl;
return 0;
}
```
代码注释如下:
```c++
#include <iostream> // 标准输入输出头文件
#include <fstream> // 文件输入输出头文件
#include <string> // 字符串头文件
using namespace std;
// 定义城市结构体
struct City {
int id; // 城市序号
string name; // 城市名称
double gdp; // 城市GDP总量(单位为亿元)
int population; // 城市常驻人口数(单位为万人)
};
int main() {
// 打开数据文件
ifstream infile("gdp_data.txt");
if (!infile) { // 判断文件是否打开成功
cout << "文件打开失败!" << endl;
return 0;
}
// 读取数据文件中的城市数据
City cities[21]; // 定义城市数组
for (int i = 0; i < 21; i++) { // 循环读取每行数据
infile >> cities[i].id >> cities[i].name >> cities[i].gdp >> cities[i].population;
}
infile.close(); // 关闭文件
// 计算每个城市的人均GDP并输出
cout << "各城市人均GDP如下:" << endl;
for (int i = 0; i < 21; i++) {
double perGdp = cities[i].gdp * 10000 / cities[i].population; // 人均GDP计算公式
cout << cities[i].name << ":" << perGdp << "元/人" << endl;
}
// 计算人均GDP的最大值和最小值以及对应城市的名字并输出
double maxPerGdp = cities[0].gdp * 10000 / cities[0].population; // 初始化最大值和最小值
double minPerGdp = cities[0].gdp * 10000 / cities[0].population;
string maxCity = cities[0].name;
string minCity = cities[0].name;
for (int i = 1; i < 21; i++) { // 从第二个城市开始循环比较
double perGdp = cities[i].gdp * 10000 / cities[i].population; // 人均GDP计算公式
if (perGdp > maxPerGdp) { // 更新最大值和对应城市名字
maxPerGdp = perGdp;
maxCity = cities[i].name;
}
if (perGdp < minPerGdp) { // 更新最小值和对应城市名字
minPerGdp = perGdp;
minCity = cities[i].name;
}
}
cout << "人均GDP最大值为:" << maxPerGdp << "元/人,对应城市为:" << maxCity << endl;
cout << "人均GDP最小值为:" << minPerGdp << "元/人,对应城市为:" << minCity << endl;
return 0;
}
```
代码中的变量和函数含义如下:
- `City`:自定义的城市结构体,包含城市序号、城市名称、城市GDP总量和城市常驻人口数四个成员变量。
- `infile`:文件输入流,用于读取数据文件。
- `cities`:城市结构体数组,用于储存数据文件中的城市数据。
- `perGdp`:当前城市的人均GDP,由城市GDP总量和常住人口数计算得出。
- `maxPerGdp`和`minPerGdp`:人均GDP的最大值和最小值。
- `maxCity`和`minCity`:人均GDP最大值和最小值对应的城市名称。
- `ifstream`:文件输入流对象,用于从文件中读取数据。
- `if (!infile)`:判断文件是否打开成功,如果失败则输出错误信息并结束程序。
- `for`循环:用于读取数据文件中的城市数据。
- `cout`:标准输出流对象,用于输出数据。
- `return`:结束程序并返回指定值。
阅读全文
相关推荐
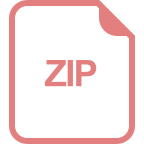
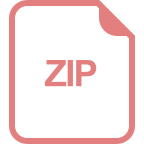
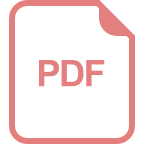
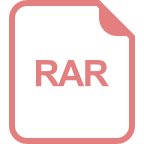
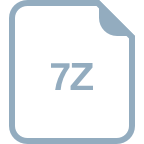
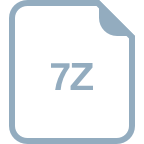
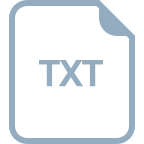