输入学生信息,包括学号成绩姓名,当学号为0时停止,建立一个单链表,当输入一个学生信息时,将输入的学生成绩与之前进行比较,输出较大的一个,用c语言
时间: 2024-12-04 22:25:05 浏览: 6
在C语言中,我们可以创建一个结构体`Student`来存储学生的相关信息,并使用一个单链表数据结构来存储并比较他们的成绩。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int id;
float score;
char name[50];
struct Student* next;
} Student;
// 初始化链表头节点
Student* createNode(int id, float score, const char* name) {
Student* newNode = (Student*)malloc(sizeof(Student));
newNode->id = id;
newNode->score = score;
strncpy(newNode->name, name, sizeof(newNode->name) - 1);
newNode->next = NULL;
return newNode;
}
// 比较并更新最大成绩
void updateMaxScore(Student** head, Student* newNode) {
if (*head == NULL || newNode->score > (*head)->score) {
Student* temp = *head;
*head = newNode;
if (temp != NULL) {
free(temp);
}
} else {
Student* current = *head;
while (current->next != NULL && current->next->score > newNode->score) {
current = current->next;
}
if (current->next && newNode->score > current->next->score) {
current->next = newNode;
}
}
}
// 添加新学生到链表
void insertStudent(Student** head, int id, float score, const char* name) {
Student* newNode = createNode(id, score, name);
if (*head == NULL) {
*head = newNode;
} else {
Student* current = *head;
while (current->next != NULL && current->id != 0) {
current = current->next;
}
if (current->id == 0) { // 如果当前节点学号为0,添加至链表尾部
current->next = newNode;
} else {
updateMaxScore(head, newNode);
}
}
}
int main() {
Student* head = NULL;
int inputId;
printf("请输入学生的学号(输入0结束):");
while (scanf("%d", &inputId), inputId != 0) {
char name[50];
float score;
printf("请输入学号 %d 的成绩和姓名:", inputId);
scanf("%f %s", &score, name);
insertStudent(&head, inputId, score, name);
}
Student* current = head;
while (current != NULL) {
printf("学号:%d, 成绩:%f, 姓名:%s\n", current->id, current->score, current->name);
current = current->next;
}
return 0;
}
```
在这个程序中,我们首先定义了一个`Student`结构体和相关的操作函数,如创建节点、更新最大成绩以及插入新学生。然后在主函数里通过循环不断读取用户输入,直到学号为0为止。
阅读全文
相关推荐
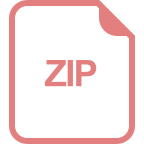
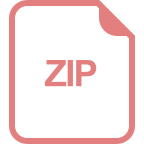
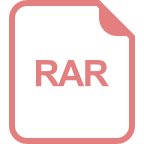


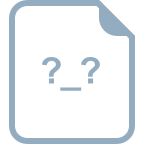
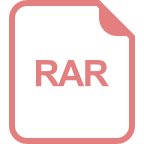
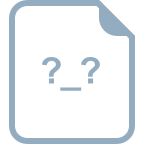
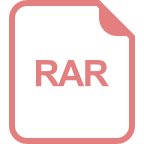
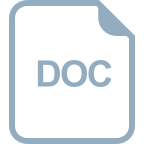
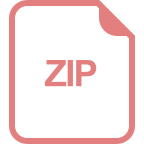







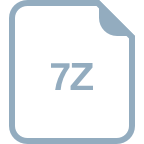