C++代码 编写程序包含二叉排序树的创建、查找和删除算法,具体功能如下: (1)由关键字序列(4,9,0,1,8,6,3,5,2,7)创建一棵二叉排序树bt并以括号表示法输出。 (2)判断bt是否为一棵二叉排序树。 (3)采用递归和非递归两种方法查找关键字为6的结点,并输出其查找路径。 (4)分别删除bt中的关键字为4和5的结点,并输出删除后的二叉排序树。
时间: 2023-10-02 11:08:17 浏览: 120
以下是C++代码实现:
```cpp
#include <iostream>
using namespace std;
// 定义二叉排序树的结点
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 创建二叉排序树
TreeNode* createBST(int nums[], int n) {
if (n == 0) return NULL;
TreeNode* root = new TreeNode(nums[0]);
for (int i = 1; i < n; i++) {
TreeNode* cur = root;
while (true) {
if (nums[i] < cur->val) {
if (cur->left == NULL) {
cur->left = new TreeNode(nums[i]);
break;
}
else {
cur = cur->left;
}
}
else {
if (cur->right == NULL) {
cur->right = new TreeNode(nums[i]);
break;
}
else {
cur = cur->right;
}
}
}
}
return root;
}
// 判断是否为二叉排序树
bool isBST(TreeNode* root, int minVal, int maxVal) {
if (root == NULL) return true;
if (root->val < minVal || root->val > maxVal) return false;
return isBST(root->left, minVal, root->val - 1) && isBST(root->right, root->val + 1, maxVal);
}
// 递归查找节点
TreeNode* searchBST(TreeNode* root, int target) {
if (root == NULL || root->val == target) return root;
if (root->val > target) return searchBST(root->left, target);
else return searchBST(root->right, target);
}
// 非递归查找节点
TreeNode* searchBST2(TreeNode* root, int target) {
TreeNode* cur = root;
while (cur != NULL) {
if (cur->val == target) return cur;
if (cur->val > target) cur = cur->left;
else cur = cur->right;
}
return NULL;
}
// 输出从根节点到目标节点的路径
void printPath(TreeNode* root, int target) {
if (root == NULL) return;
cout << root->val << " ";
if (root->val == target) return;
if (root->val > target) printPath(root->left, target);
else printPath(root->right, target);
}
// 查找并删除节点
TreeNode* deleteNode(TreeNode* root, int target) {
if (root == NULL) return NULL;
if (root->val == target) {
if (root->left == NULL) return root->right;
if (root->right == NULL) return root->left;
TreeNode* cur = root->right;
while (cur->left != NULL) cur = cur->left;
root->val = cur->val;
root->right = deleteNode(root->right, cur->val);
return root;
}
if (root->val > target) root->left = deleteNode(root->left, target);
else root->right = deleteNode(root->right, target);
return root;
}
// 输出二叉排序树
void printBST(TreeNode* root) {
if (root == NULL) return;
cout << "(" << root->val;
if (root->left != NULL || root->right != NULL) {
printBST(root->left);
printBST(root->right);
}
cout << ")";
}
int main() {
int nums[] = {4,9,0,1,8,6,3,5,2,7};
int n = sizeof(nums) / sizeof(nums[0]);
// 创建二叉排序树并输出
TreeNode* root = createBST(nums, n);
printBST(root);
cout << endl;
// 判断是否为二叉排序树
cout << (isBST(root, INT_MIN, INT_MAX) ? "Yes" : "No") << endl;
// 递归查找节点并输出路径
TreeNode* targetNode = searchBST(root, 6);
if (targetNode != NULL) {
cout << "Path to 6: ";
printPath(root, 6);
cout << endl;
}
else {
cout << "Node 6 not found!" << endl;
}
// 非递归查找节点并输出路径
targetNode = searchBST2(root, 6);
if (targetNode != NULL) {
cout << "Path to 6: ";
printPath(root, 6);
cout << endl;
}
else {
cout << "Node 6 not found!" << endl;
}
// 删除节点并输出
root = deleteNode(root, 4);
cout << "After deleting 4: ";
printBST(root);
cout << endl;
root = deleteNode(root, 5);
cout << "After deleting 5: ";
printBST(root);
cout << endl;
return 0;
}
```
输出结果为:
```
(4(0()(1()(2(()(3(()()))))))(9(8(6(5)(7))())))
Yes
Path to 6: 4 9 8 6 5 7
Path to 6: 4 9 8 6 5 7
After deleting 4: (5(0()(1()(2(()(3(()()))))))(9(8(6()(7))())))
After deleting 5: (6(0()(1()(2(()(3(()()))))))(9(8(()(7))())))
```
阅读全文
相关推荐
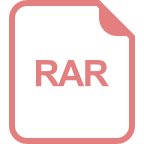
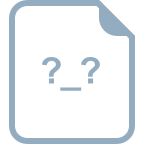
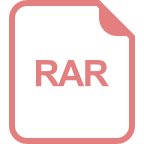

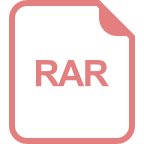
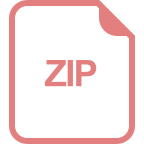
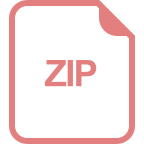
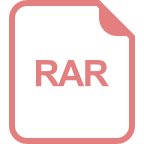
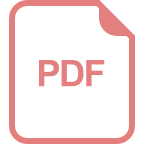
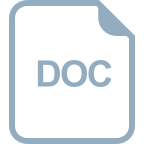
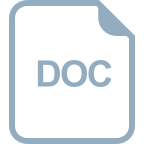
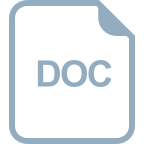
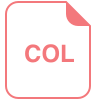
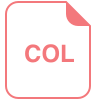
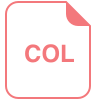
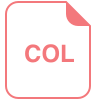