修改上述代码,不需要计算none值
时间: 2023-12-06 12:39:44 浏览: 96
下面是修改后的代码:
```python
def get_sum(nums):
total = 0
for num in nums:
if num is not None:
total += num
return total
```
这样就不会计算 None 值了。
相关问题
上述代码报memoryerror的错误,请修改上述代码,给我完整代码
由于原始代码提到的MemoryError通常是因为内存不足以一次性加载大量数据,我们可以尝试分批处理或者使用更高效的数据结构来避免这个问题。下面是一个修改后的版本,采用了pandas DataFrame分块读取和处理的方式,这样可以减少内存消耗:
```python
import pandas as pd
import numpy as np
from itertools import islice
import openpyxl
import networkx as nx
# 假设grouped是已经按某种方式分组的数据,例如DataFrame的GroupBy对象
# 或者是列表的列表,每一层代表一组数据
def process_group_in_chunks(grouped, max_memory_usage, feature_df, feature_weights_df, common_matrix, chunksize=1000):
for i, group in enumerate(islice(grouped, 0, None, chunksize)):
feature_words_chunk = feature_df.iloc[i:i+chunksize]
feature_weights_chunk = feature_weights_df.iloc[i:i+chunksize]
common_matrix_chunk = common_matrix[i:i+chunksize]
# 对每一块数据进行操作
num_features = len(feature_words_chunk)
for j in range(num_features):
# ... (其他操作,如创建Excel和Graph)
# 保存当前组的数据后,释放内存
del feature_words_chunk
del feature_weights_chunk
del common_matrix_chunk
# 原始数据假设存储在dataframes feature_df, feature_weights_df, 和 common_matrix中
grouped_data = ... # 填充实际数据源
max_memory_usage = ... # 根据你的系统内存设定合适的最大内存使用量
# 分块处理数据
process_group_in_chunks(grouped_data, max_memory_usage, feature_df, feature_weights_df, common_matrix)
```
在这个版本中,我们使用了`islice`函数按块处理`grouped`数据,每次只加载一部分到内存,完成计算后再释放这部分数据,降低内存压力。这样就可以避免因为数据过大导致的MemoryError。
修改上述代码:调用摄像头进行实时处理
要将上述代码从读取视频文件修改为使用摄像头进行实时处理,可以按照以下步骤进行调整:
1. **初始化摄像头**:使用 `cv2.VideoCapture` 捕获摄像头输入。
2. **移除与视频文件相关的部分**:例如,获取帧数和设置输出视频等。
3. **实时处理每一帧**:在循环中读取摄像头帧并进行处理。
以下是修改后的代码:
```python
import numpy as np
import cv2
# 初始化摄像头
cap = cv2.VideoCapture(0)
# 获取摄像头图像的宽度和高度
w = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
h = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# 定义平滑半径
SMOOTHING_RADIUS = 50
# 读取第一帧
success, prev = cap.read()
if not success:
raise Exception("Failed to read the first frame")
# 转换为灰度图
prev_gray = cv2.cvtColor(prev, cv2.COLOR_BGR2GRAY)
# 预定义变换存储数组
transforms = []
while True:
# 检测前一帧中的特征点
prev_pts = cv2.goodFeaturesToTrack(prev_gray, maxCorners=200, qualityLevel=0.01, minDistance=30, blockSize=3)
# 读取下一帧
success, curr = cap.read()
if not success:
break
# 转换为灰度图
curr_gray = cv2.cvtColor(curr, cv2.COLOR_BGR2GRAY)
# 计算光流(即跟踪特征点)
curr_pts, status, err = cv2.calcOpticalFlowPyrLK(prev_gray, curr_gray, prev_pts, None)
# 检查有效性
assert prev_pts.shape == curr_pts.shape
# 过滤只有有效点
idx = np.where(status == 1)[0]
prev_pts = prev_pts[idx]
curr_pts = curr_pts[idx]
# 计算变换矩阵
m = cv2.estimateRigidTransform(prev_pts, curr_pts, fullAffine=False) # 仅适用于OpenCV-3或更早版本
if m is not None:
# 提取平移
dx = m[0, 2]
dy = m[1, 2]
# 提取旋转角度
da = np.arctan2(m[1, 0], m[0, 0])
# 存储变换
transforms.append([dx, dy, da])
else:
transforms.append([0, 0, 0])
# 移动到下一帧
prev_gray = curr_gray
# 打印当前帧的信息
print(f"Frame: {len(transforms)} - Tracked points: {len(prev_pts)}")
# 如果按下q键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 关闭摄像头
cap.release()
# 计算轨迹
trajectory = np.cumsum(transforms, axis=0)
# 平滑轨迹
smoothed_trajectory = smooth(trajectory)
# 计算新的变换数组
transforms_smooth = transforms + (smoothed_trajectory - trajectory)
# 重新初始化摄像头
cap = cv2.VideoCapture(0)
# 读取第一帧
success, prev = cap.read()
if not success:
raise Exception("Failed to read the first frame")
# 转换为灰度图
prev_gray = cv2.cvtColor(prev, cv2.COLOR_BGR2GRAY)
# 实时稳定化处理
while True:
# 读取下一帧
success, frame = cap.read()
if not success:
break
# 提取变换
dx = transforms_smooth[len(transforms_smooth) - len(transforms) - 1][0]
dy = transforms_smooth[len(transforms_smooth) - len(transforms) - 1][1]
da = transforms_smooth[len(transforms_smooth) - len(transforms) - 1][2]
# 重构变换矩阵
m = np.zeros((2, 3), np.float32)
m[0, 0] = np.cos(da)
m[0, 1] = -np.sin(da)
m[1, 0] = np.sin(da)
m[1, 1] = np.cos(da)
m[0, 2] = dx
m[1, 2] = dy
# 应用仿射变换
frame_stabilized = cv2.warpAffine(frame, m, (w, h))
# 固定边界伪影
frame_stabilized = fixBorder(frame_stabilized)
# 将原始帧和稳定化后的帧拼接在一起显示
frame_out = cv2.hconcat([frame, frame_stabilized])
# 如果图像太大,缩小尺寸
if frame_out.shape[1] > 1920:
frame_out = cv2.resize(frame_out, (frame_out.shape[1] // 2, frame_out.shape[0] // 2))
# 显示结果
cv2.imshow("Before and After", frame_out)
# 如果按下q键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
def movingAverage(curve, radius):
window_size = 2 * radius + 1
f = np.ones(window_size) / window_size
curve_pad = np.lib.pad(curve, (radius, radius), 'edge')
curve_smoothed = np.convolve(curve_pad, f, mode='same')
curve_smoothed = curve_smoothed[radius:-radius]
return curve_smoothed
def smooth(trajectory):
smoothed_trajectory = np.copy(trajectory)
for i in range(3):
smoothed_trajectory[:, i] = movingAverage(trajectory[:, i], radius=SMOOTHING_RADIUS)
return smoothed_trajectory
def fixBorder(frame):
s = frame.shape
T = cv2.getRotationMatrix2D((s[1] / 2, s[0] / 2), 0, 1.04)
frame = cv2.warpAffine(frame, T, (s[1], s[0]))
return frame
```
### 主要修改点:
1. **初始化摄像头**:使用 `cv2.VideoCapture(0)` 捕获摄像头输入。
2. **移除与视频文件相关的部分**:如获取帧数、设置输出视频等。
3. **实时处理每一帧**:在主循环中读取摄像头帧并进行处理。
4. **平滑处理**:计算轨迹并进行平滑处理后,重新初始化摄像头进行稳定化处理。
这样,代码就可以实现实时从摄像头捕获视频并进行稳定化处理。
阅读全文
相关推荐
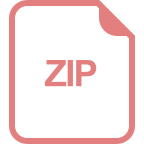
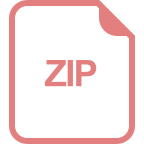
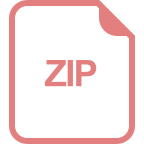












