电影租赁sql数据进行数据挖掘代码
时间: 2024-03-21 17:41:51 浏览: 11
首先,我们需要准备一个包含电影租赁信息的 SQL 数据库。下面是一个简单的示例:
```
CREATE TABLE customers (
id INT PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255)
);
CREATE TABLE movies (
id INT PRIMARY KEY,
title VARCHAR(255),
year INT,
genre VARCHAR(255)
);
CREATE TABLE rentals (
id INT PRIMARY KEY,
customer_id INT,
movie_id INT,
rented_on DATE,
returned_on DATE,
rental_fee DECIMAL(5, 2),
late_fee DECIMAL(5, 2)
);
```
这个数据库包含三个表格:`customers`、`movies`和`rentals`。`customers`表格包含客户信息,`movies`表格包含电影信息,`rentals`表格包含租赁信息。
接下来,我们可以使用以下 Python 代码连接数据库并进行数据挖掘:
```python
import mysql.connector
import pandas as pd
import matplotlib.pyplot as plt
# 连接数据库
cnx = mysql.connector.connect(user='username', password='password',
host='localhost',
database='rental')
# 从数据库中读取数据
customers_df = pd.read_sql('SELECT * FROM customers', con=cnx)
movies_df = pd.read_sql('SELECT * FROM movies', con=cnx)
rentals_df = pd.read_sql('SELECT * FROM rentals', con=cnx)
# 分析数据
# 统计每个电影的租借次数
movie_rental_counts = rentals_df.groupby('movie_id')['id'].count()
movie_rental_counts = movie_rental_counts.reset_index()
movie_rental_counts.columns = ['movie_id', 'rental_count']
movie_rental_counts = pd.merge(movie_rental_counts, movies_df, on='movie_id')
movie_rental_counts = movie_rental_counts.sort_values(by='rental_count', ascending=False)
# 统计每个客户的租借次数
customer_rental_counts = rentals_df.groupby('customer_id')['id'].count()
customer_rental_counts = customer_rental_counts.reset_index()
customer_rental_counts.columns = ['customer_id', 'rental_count']
customer_rental_counts = pd.merge(customer_rental_counts, customers_df, on='customer_id')
customer_rental_counts = customer_rental_counts.sort_values(by='rental_count', ascending=False)
# 统计每个电影的平均租借费用和逾期费用
movie_rental_fees = rentals_df.groupby('movie_id')['rental_fee', 'late_fee'].mean()
movie_rental_fees = movie_rental_fees.reset_index()
movie_rental_fees.columns = ['movie_id', 'avg_rental_fee', 'avg_late_fee']
movie_rental_fees = pd.merge(movie_rental_fees, movies_df, on='movie_id')
# 可视化数据
# 绘制电影租借次数排名前10的电影柱状图
fig, ax = plt.subplots()
movie_rental_counts.head(10).plot(kind='bar', x='title', y='rental_count', ax=ax)
ax.set_title('Top 10 Movies by Rental Count')
ax.set_xlabel('Movie Title')
ax.set_ylabel('Rental Count')
plt.show()
# 绘制客户租借次数排名前10的客户柱状图
fig, ax = plt.subplots()
customer_rental_counts.head(10).plot(kind='bar', x='name', y='rental_count', ax=ax)
ax.set_title('Top 10 Customers by Rental Count')
ax.set_xlabel('Customer Name')
ax.set_ylabel('Rental Count')
plt.show()
# 绘制不同电影的平均租借费用和逾期费用散点图
fig, ax = plt.subplots()
movie_rental_fees.plot(kind='scatter', x='avg_rental_fee', y='avg_late_fee', ax=ax)
ax.set_title('Rental Fees by Movie')
ax.set_xlabel('Average Rental Fee')
ax.set_ylabel('Average Late Fee')
plt.show()
# 关闭数据库连接
cnx.close()
```
这个代码使用了 Pandas 和 Matplotlib 库来进行数据挖掘和可视化。我们首先连接数据库,然后从数据库中读取客户、电影和租赁信息。接下来,我们对数据进行了一些分析,比如统计每个电影和客户的租借次数,以及每个电影的平均租借费用和逾期费用。最后,我们使用 Matplotlib 库绘制了柱状图和散点图来展示数据。
相关推荐
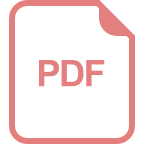














