中断接收的消息,缓存在消息队列中
时间: 2024-03-12 20:49:26 浏览: 21
在使用消息队列进行进程间通信的时候,我们可以使用信号来中断进程的运行,然后将中断接收的消息缓存在消息队列中,等待处理完中断后再进行处理。
下面是一个简单的示例代码,实现了在接收到 SIGUSR1 信号时,将消息缓存在消息队列中。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <signal.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 128
typedef struct msgbuf
{
long mtype;
char mtext[MSG_SIZE];
} MsgBuf;
MsgBuf msg;
void sig_handler(int signo)
{
int msqid;
key_t key;
// 打开消息队列
key = ftok(".", 'a');
msqid = msgget(key, IPC_CREAT | 0666);
if(msqid == -1)
{
perror("msgget");
exit(1);
}
// 将消息缓存到消息队列
msg.mtype = 1;
strcpy(msg.mtext, "Interrupted message!");
msgsnd(msqid, &msg, strlen(msg.mtext)+1, 0);
}
int main()
{
// 注册信号处理函数
signal(SIGUSR1, sig_handler);
// 等待信号
while(1)
{
pause();
}
return 0;
}
```
这个示例中,我们注册了一个 SIGUSR1 信号的处理函数 sig_handler,当接收到这个信号时,就将消息缓存在消息队列中。在主函数中,我们使用 pause 函数等待信号的到来,一旦接收到信号就会进入到信号处理函数中。
需要注意的是,这个示例中只缓存了一条消息,如果需要缓存多条消息,可以使用循环等待信号,并将每条中断接收的消息缓存到消息队列中。同时,为了保证消息队列的安全性,我们需要对消息队列进行锁定,以避免多个进程同时访问造成的竞争问题。
相关推荐
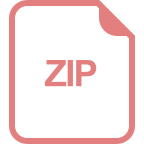
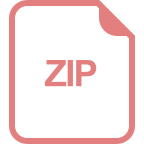
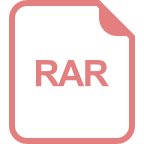














