Person类的结构如图所示,包含姓名,身份证号,性别,年龄四个成员数据,以及构造函数,析构函数,显示信息函数。 要求在此基础上派生出Employee类,该类新增两个数据成员,分别为部门和薪水,Employee包含构造函数,析构函数,显示函数。 请1)完成Person类的各成员函数,2)完成Employee类的定义以及Employee各成员函数的定义。 class Person{ protected: char *name; char *id; int sex; ///0:女,1:男 int age; public: Person(char *p_name,char *p_id,int p_sex,int p_age); void show(); ~Person(); };
时间: 2024-03-12 22:45:24 浏览: 91
1)完成Person类的各成员函数:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class Person{
protected:
char *name;
char *id;
int sex; // 0:女,1:男
int age;
public:
Person(char *p_name,char *p_id,int p_sex,int p_age){
name = new char[strlen(p_name)+1];
strcpy(name, p_name);
id = new char[strlen(p_id)+1];
strcpy(id, p_id);
sex = p_sex;
age = p_age;
}
void show(){
cout<<"姓名:"<<name<<endl;
cout<<"身份证号:"<<id<<endl;
cout<<"性别:"<< (sex==1?"男":"女") <<endl;
cout<<"年龄:"<<age<<endl;
}
~Person(){
delete[] name;
delete[] id;
}
};
```
2)完成Employee类的定义以及Employee各成员函数的定义:
```cpp
class Employee: public Person{
private:
char *department;
int salary;
public:
Employee(char *p_name,char *p_id,int p_sex,int p_age, char *p_department, int p_salary)
:Person(p_name, p_id, p_sex, p_age){
department = new char[strlen(p_department)+1];
strcpy(department, p_department);
salary = p_salary;
}
void show(){
Person::show();
cout<<"部门:"<<department<<endl;
cout<<"薪水:"<<salary<<endl;
}
~Employee(){
delete[] department;
}
};
```
阅读全文
相关推荐
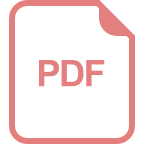
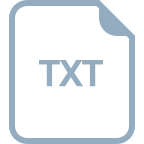
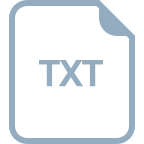















