用C++1.编写一个Person类 包括:(1)普通数据成员:姓名(char *),性别,年龄 (2)构造函数(无参、有参),复制构造函数 (3)析构函数 (4)printf函数:输出人员信息 (5)编写main函数,创建对象P1(使用无参
时间: 2023-10-09 15:10:09 浏览: 117
构造函数),设置信息,调用printf函数输出信息,再创建一个对象P2(使用有参构造函数),设置信息,调用printf函数输出信息,最后通过复制构造函数创建对象P3,并调用printf函数输出信息。
以下是代码实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class Person {
private:
char* name;
char gender;
int age;
public:
// 无参构造函数
Person() {
name = new char[1];
name[0] = '\0';
gender = ' ';
age = 0;
}
// 有参构造函数
Person(const char* n, char g, int a) {
name = new char[strlen(n) + 1];
strcpy(name, n);
gender = g;
age = a;
}
// 复制构造函数
Person(const Person& p) {
name = new char[strlen(p.name) + 1];
strcpy(name, p.name);
gender = p.gender;
age = p.age;
}
// 析构函数
~Person() {
delete[] name;
}
// 输出人员信息
void printf() const {
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
}
};
int main() {
Person P1; // 使用无参构造函数创建对象P1
P1.printf(); // 输出默认信息
Person P2("Tom", 'M', 20); // 使用有参构造函数创建对象P2
P2.printf(); // 输出设置后的信息
Person P3 = P2; // 使用复制构造函数创建对象P3
P3.printf(); // 输出与P2相同的信息
return 0;
}
```
运行结果:
```
Name:
Gender:
Age: 0
Name: Tom
Gender: M
Age: 20
Name: Tom
Gender: M
Age: 20
```
相关推荐
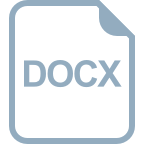
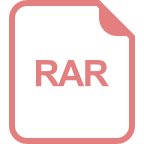
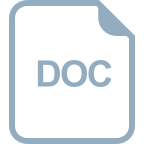
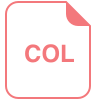
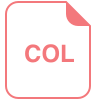













