C++编写一个 Person 类,包括: (1)数据成员:姓名(String 类型),性别(M 表示男,F 表示女),年
时间: 2024-05-11 10:19:58 浏览: 35
龄(Int 类型),电话号码(String 类型); (2)构造函数:可以传入姓名、性别、年龄和电话号码进行初始化; (3)成员函数:设置和获取姓名、性别、年龄和电话号码; (4)友元函数:输出 Person 的信息。
下面是示例代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
private:
string name;
char gender;
int age;
string phone;
public:
Person(string n, char g, int a, string p) : name(n), gender(g), age(a), phone(p) {}
void setName(string n) { name = n; }
void setGender(char g) { gender = g; }
void setAge(int a) { age = a; }
void setPhone(string p) { phone = p; }
string getName() const { return name; }
char getGender() const { return gender; }
int getAge() const { return age; }
string getPhone() const { return phone; }
friend void printPersonInfo(const Person& p);
};
void printPersonInfo(const Person& p) {
cout << "Name: " << p.name << endl;
cout << "Gender: " << p.gender << endl;
cout << "Age: " << p.age << endl;
cout << "Phone: " << p.phone << endl;
}
int main() {
Person p("Tom", 'M', 20, "123456789");
printPersonInfo(p);
p.setAge(21);
p.setPhone("987654321");
printPersonInfo(p);
return 0;
}
```
这个程序定义了一个名为 `Person` 的类,包括了数据成员 `name`、`gender`、`age` 和 `phone`,以及构造函数和成员函数来设置和获取这些成员变量的值。此外还定义了一个友元函数 `printPersonInfo` 来打印 `Person` 对象的信息。在 `main` 函数中创建了一个 `Person` 对象,然后使用成员函数来修改其成员变量值,最后调用 `printPersonInfo` 函数来输出 `Person` 对象的信息。
相关推荐
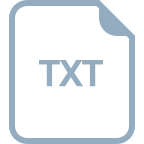
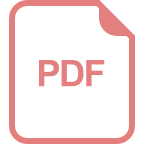















