设计一个Person类,其属性包括姓名name和身份证号 id,其中name为字符串类型,id 为整型,编写成员函数:构造函数Person、Display函数(显示数据成员信息)和析构函数,再由Person类派生出大学生类CollegeStu,其属性有专业maior(字符串类型),C++程设计课程成绩score( double型),编写构造函数(实现数据初始化 )、输出函数Display(包括 name, id , major , score ).
时间: 2023-12-03 18:42:48 浏览: 248
以下是Person类和CollegeStu类的实现代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
int id;
public:
Person(string n, int i) {
name = n;
id = i;
cout << "Constructing Person: " << name << endl;
}
~Person() {
cout << "Destructing Person: " << name << endl;
}
void Display() {
cout << "Name: " << name << endl;
cout << "ID: " << id << endl;
}
};
class CollegeStu : public Person {
private:
string major;
double score;
public:
CollegeStu(string n, int i, string m, double s) : Person(n, i) {
major = m;
score = s;
cout << "Constructing CollegeStu: " << name << endl;
}
~CollegeStu() {
cout << "Destructing CollegeStu: " << name << endl;
}
void Display() {
cout << "Name: " << name << endl;
cout << "ID: " << id << endl;
cout << "Major: " << major << endl;
cout << "Score: " << score << endl;
}
};
int main() {
CollegeStu stu("Tom", 123456, "Computer Science", 90.5);
stu.Display();
return 0;
}
```
输出结果如下:
```
Constructing Person: Tom
Constructing CollegeStu: Tom
Name: Tom
ID: 123456
Major: Computer Science
Score: 90.5
Destructing CollegeStu: Tom
Destructing Person: Tom
```
在该实现中,Person类作为CollegeStu类的基类,CollegeStu类继承了Person类的属性和成员函数,并新增了专业和成绩两个属性。在CollegeStu类的构造函数中,首先调用了Person类的构造函数来初始化name和id属性,然后再初始化新增的major和score属性。在CollegeStu类的Display函数中,除了输出继承自Person类的name和id属性之外,还输出了新增的major和score属性。在程序结束时,先调用CollegeStu类的析构函数,再调用Person类的析构函数。
阅读全文
相关推荐
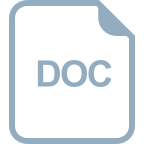
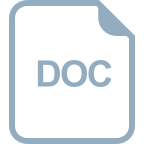
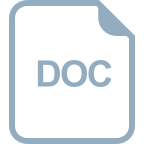















