构建一个类Person,包含字符串成员name(姓名),整型数据成员age(年龄),成员函数 display()用来输出name和age。构造函数包含两个参数,用来对name和age初始化。构建一个类Employee由Person派生,包含department(部门),实型数据成员salary(工资),成员函数display()用来输出职工姓名、年龄、部门、工资,其他成员根据需要自己设定。主函数中定义3个Employee类对象,内容自己设定,将其姓名、年龄、部门、工资输出,并计算他们的平均工资。
时间: 2024-04-11 12:26:53 浏览: 56
以下是根据您的要求构建的Person和Employee类的示例代码:
```cpp
#include <iostream>
#include <string>
class Person {
protected:
std::string name;
int age;
public:
Person(const std::string& _name, int _age)
: name(_name), age(_age) {}
void display() {
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
}
};
class Employee : public Person {
private:
std::string department;
double salary;
public:
Employee(const std::string& _name, int _age, const std::string& _department, double _salary)
: Person(_name, _age), department(_department), salary(_salary) {}
void display() {
Person::display();
std::cout << "Department: " << department << std::endl;
std::cout << "Salary: " << salary << std::endl;
}
double getSalary() {
return salary;
}
};
int main() {
Employee employee1("John Doe", 30, "IT", 5000.0);
Employee employee2("Jane Smith", 35, "HR", 6000.0);
Employee employee3("Mike Johnson", 28, "Sales", 5500.0);
employee1.display();
std::cout << std::endl;
employee2.display();
std::cout << std::endl;
employee3.display();
std::cout << std::endl;
// 计算平均工资
double averageSalary = (employee1.getSalary() + employee2.getSalary() + employee3.getSalary()) / 3.0;
std::cout << "Average Salary: " << averageSalary << std::endl;
return 0;
}
```
在主函数中,我们创建了3个Employee对象,并按要求输出了他们的姓名、年龄、部门和工资。最后,我们计算了这三个员工的平均工资并进行输出。
相关推荐
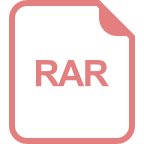
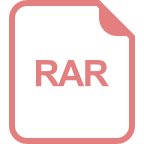
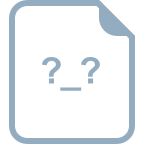















