C++编写一个学生和教师的数据输入和显示程序。学生数据有编号、姓名、性别、年龄、系别和成绩,教师数据有编号、姓名、性别、年龄、职称和部门。要求将编号、姓名、性别、年龄的输入和显示设计成一个类Person,并作为学生类Student和教师类Teacher的基类
时间: 2023-12-22 22:20:06 浏览: 80
以下是一个简单的C++程序,实现了学生和教师的数据输入和显示,其中Person类作为学生类和教师类的基类。
```c++
#include<iostream>
#include<string>
using namespace std;
class Person {
protected:
int id;
string name;
char gender;
int age;
public:
Person(int i, string n, char g, int a) : id(i), name(n), gender(g), age(a) {}
virtual void display() {
cout << "编号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
}
};
class Student : public Person {
private:
string department;
double score;
public:
Student(int i, string n, char g, int a, string d, double s)
: Person(i, n, g, a), department(d), score(s) {}
void display() {
Person::display();
cout << "系别:" << department << endl;
cout << "成绩:" << score << endl;
}
};
class Teacher : public Person {
private:
string title;
string department;
public:
Teacher(int i, string n, char g, int a, string t, string d)
: Person(i, n, g, a), title(t), department(d) {}
void display() {
Person::display();
cout << "职称:" << title << endl;
cout << "部门:" << department << endl;
}
};
int main() {
Student s(1001, "张三", 'M', 20, "计算机科学与技术", 90);
Teacher t(2001, "李老师", 'F', 30, "副教授", "计算机系");
s.display();
cout << endl;
t.display();
return 0;
}
```
程序输出结果为:
```
编号:1001
姓名:张三
性别:M
年龄:20
系别:计算机科学与技术
成绩:90
编号:2001
姓名:李老师
性别:F
年龄:30
职称:副教授
部门:计算机系
```
阅读全文
相关推荐
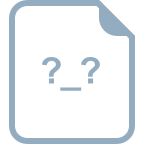
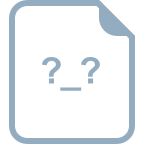



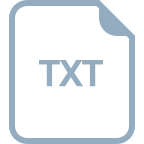
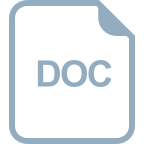
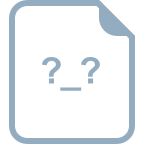
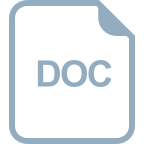
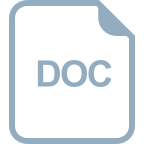
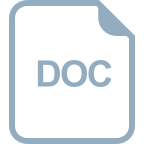
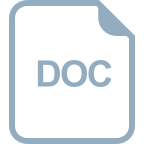
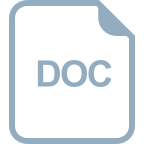
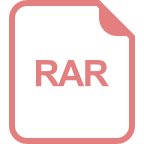
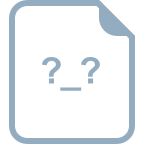
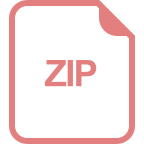