java FileChannel高并发处理
时间: 2023-09-06 08:08:58 浏览: 132
在Java中,可以使用FileChannel来实现高并发处理。FileChannel提供了一种直接操作文件的方式,可以在多个线程之间共享文件,并实现高效的读写操作。下面是一个示例代码,展示如何使用FileChannel进行高并发处理:
```java
import java.io.*;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.*;
public class FileProcessing {
public static void main(String[] args) {
String filePath = "path/to/file";
try {
// 打开文件通道
FileChannel channel = FileChannel.open(Paths.get(filePath), StandardOpenOption.READ);
// 创建多个线程同时读取文件
int threadCount = 5;
for (int i = 0; i < threadCount; i++) {
Thread thread = new Thread(() -> {
try {
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (channel.read(buffer) != -1) {
buffer.flip(); // 切换为读模式
// 处理文件内容
// ...
buffer.clear(); // 清空缓冲区,为下一次读取做准备
}
} catch (IOException e) {
e.printStackTrace();
}
});
thread.start();
}
// 等待所有线程执行完毕
for (int i = 0; i < threadCount; i++) {
Thread.currentThread().join();
}
// 关闭文件通道
channel.close();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们首先打开了一个文件通道。然后,创建了多个线程,每个线程都会读取文件通道中的内容并进行处理。在处理完一段内容后,我们需要调用`buffer.clear()`方法来清空缓冲区,为下一次读取做准备。
请注意,上述代码仅展示了如何使用FileChannel进行高并发处理的基本思路。实际应用中,你可能需要根据具体需求进行适当的调整和优化。
希望这可以帮助到你!如果你还有其他问题,请随时提问。
阅读全文
相关推荐




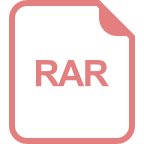













