Python使用tkinter实现计算器
时间: 2023-07-11 17:08:00 浏览: 99
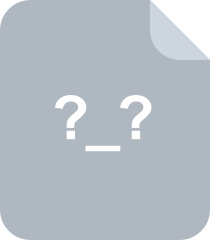
用python的tkinter库实现简易计算器
以下是一个简单的基于Tkinter的计算器实现示例:
```python
import tkinter as tk
class Calculator:
def __init__(self):
self.root = tk.Tk()
self.root.title("Calculator")
# 创建显示面板
self.display = tk.Entry(self.root, width=20, font=('Arial', 16))
self.display.grid(row=0, column=0, columnspan=4, pady=10)
# 添加按钮
# 第一行
btn_clear = tk.Button(self.root, text='C', width=5, height=2, command=self.clear)
btn_clear.grid(row=1, column=0)
btn_divide = tk.Button(self.root, text='/', width=5, height=2, command=lambda: self.button_click('/'))
btn_divide.grid(row=1, column=1)
btn_multiply = tk.Button(self.root, text='*', width=5, height=2, command=lambda: self.button_click('*'))
btn_multiply.grid(row=1, column=2)
btn_delete = tk.Button(self.root, text='DEL', width=5, height=2, command=self.delete)
btn_delete.grid(row=1, column=3)
# 第二行
btn_7 = tk.Button(self.root, text='7', width=5, height=2, command=lambda: self.button_click('7'))
btn_7.grid(row=2, column=0)
btn_8 = tk.Button(self.root, text='8', width=5, height=2, command=lambda: self.button_click('8'))
btn_8.grid(row=2, column=1)
btn_9 = tk.Button(self.root, text='9', width=5, height=2, command=lambda: self.button_click('9'))
btn_9.grid(row=2, column=2)
btn_minus = tk.Button(self.root, text='-', width=5, height=2, command=lambda: self.button_click('-'))
btn_minus.grid(row=2, column=3)
# 第三行
btn_4 = tk.Button(self.root, text='4', width=5, height=2, command=lambda: self.button_click('4'))
btn_4.grid(row=3, column=0)
btn_5 = tk.Button(self.root, text='5', width=5, height=2, command=lambda: self.button_click('5'))
btn_5.grid(row=3, column=1)
btn_6 = tk.Button(self.root, text='6', width=5, height=2, command=lambda: self.button_click('6'))
btn_6.grid(row=3, column=2)
btn_plus = tk.Button(self.root, text='+', width=5, height=2, command=lambda: self.button_click('+'))
btn_plus.grid(row=3, column=3)
# 第四行
btn_1 = tk.Button(self.root, text='1', width=5, height=2, command=lambda: self.button_click('1'))
btn_1.grid(row=4, column=0)
btn_2 = tk.Button(self.root, text='2', width=5, height=2, command=lambda: self.button_click('2'))
btn_2.grid(row=4, column=1)
btn_3 = tk.Button(self.root, text='3', width=5, height=2, command=lambda: self.button_click('3'))
btn_3.grid(row=4, column=2)
btn_equal = tk.Button(self.root, text='=', width=5, height=2, command=self.calculate)
btn_equal.grid(row=4, column=3)
# 第五行
btn_0 = tk.Button(self.root, text='0', width=5, height=2, command=lambda: self.button_click('0'))
btn_0.grid(row=5, column=0)
btn_dot = tk.Button(self.root, text='.', width=5, height=2, command=lambda: self.button_click('.'))
btn_dot.grid(row=5, column=1)
btn_left = tk.Button(self.root, text='(', width=5, height=2, command=lambda: self.button_click('('))
btn_left.grid(row=5, column=2)
btn_right = tk.Button(self.root, text=')', width=5, height=2, command=lambda: self.button_click(')'))
btn_right.grid(row=5, column=3)
def run(self):
self.root.mainloop()
def button_click(self, char):
self.display.insert(tk.END, char)
def clear(self):
self.display.delete(0, tk.END)
def delete(self):
self.display.delete(len(self.display.get()) - 1)
def calculate(self):
try:
result = eval(self.display.get())
self.display.delete(0, tk.END)
self.display.insert(tk.END, result)
except:
self.display.delete(0, tk.END)
self.display.insert(tk.END, "Error")
if __name__ == '__main__':
calculator = Calculator()
calculator.run()
```
这个实现非常基础,只支持基本的加减乘除和括号运算,并且没有做输入安全性检查,仅供参考。
阅读全文
相关推荐
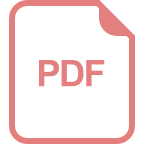


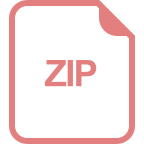
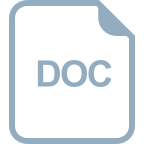
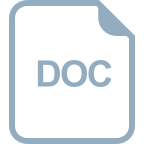




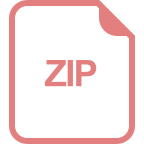
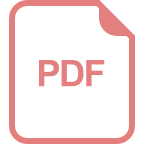
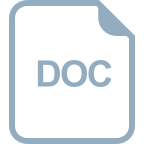
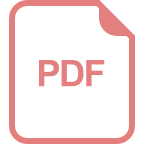
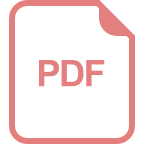