python pyqt5图像分类系统
时间: 2023-10-10 09:04:42 浏览: 112
PyQt5是一个用于开发GUI应用程序的Python库,可以与深度学习框架(如TensorFlow、PyTorch等)结合使用,实现图像分类系统。以下是实现步骤:
1. 使用PyQt5创建GUI界面,包括文件选择按钮、图像显示区域和分类结果显示区域。
2. 使用深度学习框架加载已经训练好的模型,例如ResNet、VGG等。
3. 使用OpenCV或Pillow等库读取用户选择的图像,并将其转化为模型可接受的格式。
4. 使用模型对图像进行分类,并返回分类结果。
5. 将分类结果显示在GUI界面的分类结果显示区域。
以下是代码示例(使用TensorFlow和OpenCV):
```python
import tensorflow as tf
import cv2
from PyQt5.QtWidgets import QApplication, QLabel, QMainWindow, QPushButton, QFileDialog
class ImageClassifier(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(100, 100, 640, 480)
self.setWindowTitle('Image Classifier')
self.file_button = QPushButton('Select Image', self)
self.file_button.move(20, 20)
self.file_button.clicked.connect(self.open_file_dialog)
self.image_label = QLabel(self)
self.image_label.move(20, 60)
self.result_label = QLabel(self)
self.result_label.move(20, 280)
self.show()
def open_file_dialog(self):
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_name, _ = QFileDialog.getOpenFileName(self, 'Select Image', '', 'Image Files (*.png *.jpg *.jpeg)', options=options)
if file_name:
self.show_image(file_name)
def show_image(self, file_name):
image = cv2.imread(file_name)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (224, 224))
image = image / 255.0
image = tf.expand_dims(image, axis=0)
model = tf.keras.applications.ResNet50()
result = model.predict(image)
label = tf.keras.applications.resnet.decode_predictions(result, top=1)[0][0][1]
self.result_label.setText(label)
height, width, channel = image.shape
bytes_per_line = channel * width
q_image = QImage(image.data, width, height, bytes_per_line, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(q_image)
self.image_label.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication([])
ic = ImageClassifier()
app.exec_()
```
注意:以上示例代码仅为演示用途,实际应用需要考虑更多细节和异常情况。
相关推荐
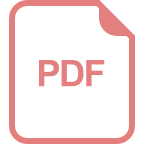
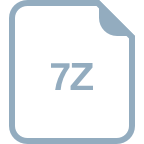














