java 写一个SM4加密解密代码
时间: 2023-08-10 10:05:26 浏览: 131
以下是 Java 实现的 SM4 加密解密的代码:
```java
import java.security.SecureRandom;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import org.apache.commons.codec.binary.Base64;
public class SM4Utils {
private String secretKey = ""; // 密钥
private String iv = ""; // 向量
private boolean hexString = false;
public SM4Utils() {
}
public SM4Utils(String secretKey) {
this.secretKey = secretKey;
this.iv = secretKey.substring(0, 16);
}
public SM4Utils(String secretKey, String iv) {
this.secretKey = secretKey;
this.iv = iv;
}
public void setHexString(boolean hexString) {
this.hexString = hexString;
}
public String encryptData_ECB(String plainText) throws Exception {
byte[] keyBytes = hexString ? Base64.decodeBase64(secretKey) : secretKey.getBytes("UTF-8");
byte[] plainBytes = hexString ? Base64.decodeBase64(plainText) : plainText.getBytes("UTF-8");
Cipher cipher = Cipher.getInstance("SM4/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, new SecretKeySpec(keyBytes, "SM4"));
byte[] encryptedBytes = cipher.doFinal(plainBytes);
return hexString ? Base64.encodeBase64String(encryptedBytes) : new String(encryptedBytes, "UTF-8");
}
public String decryptData_ECB(String cipherText) throws Exception {
byte[] keyBytes = hexString ? Base64.decodeBase64(secretKey) : secretKey.getBytes("UTF-8");
byte[] encryptedBytes = hexString ? Base64.decodeBase64(cipherText) : cipherText.getBytes("UTF-8");
Cipher cipher = Cipher.getInstance("SM4/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(keyBytes, "SM4"));
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return hexString ? Base64.encodeBase64String(decryptedBytes) : new String(decryptedBytes, "UTF-8");
}
public String encryptData_CBC(String plainText) throws Exception {
byte[] keyBytes = hexString ? Base64.decodeBase64(secretKey) : secretKey.getBytes("UTF-8");
byte[] plainBytes = hexString ? Base64.decodeBase64(plainText) : plainText.getBytes("UTF-8");
byte[] ivBytes = hexString ? Base64.decodeBase64(iv) : iv.getBytes("UTF-8");
Cipher cipher = Cipher.getInstance("SM4/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, new SecretKeySpec(keyBytes, "SM4"), new IvParameterSpec(ivBytes));
byte[] encryptedBytes = cipher.doFinal(plainBytes);
return hexString ? Base64.encodeBase64String(encryptedBytes) : new String(encryptedBytes, "UTF-8");
}
public String decryptData_CBC(String cipherText) throws Exception {
byte[] keyBytes = hexString ? Base64.decodeBase64(secretKey) : secretKey.getBytes("UTF-8");
byte[] encryptedBytes = hexString ? Base64.decodeBase64(cipherText) : cipherText.getBytes("UTF-8");
byte[] ivBytes = hexString ? Base64.decodeBase64(iv) : iv.getBytes("UTF-8");
Cipher cipher = Cipher.getInstance("SM4/CBC/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(keyBytes, "SM4"), new IvParameterSpec(ivBytes));
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return hexString ? Base64.encodeBase64String(decryptedBytes) : new String(decryptedBytes, "UTF-8");
}
public static void main(String[] args) throws Exception {
SM4Utils sm4 = new SM4Utils("1234567890123456");
String plainText = "Hello, world!";
String cipherText = sm4.encryptData_CBC(plainText);
String decryptedText = sm4.decryptData_CBC(cipherText);
System.out.println("Plain Text: " + plainText);
System.out.println("Cipher Text: " + cipherText);
System.out.println("Decrypted Text: " + decryptedText);
}
}
```
其中,`SM4Utils` 类包含了 ECB 和 CBC 两种模式的加密解密方法,可以根据需要选择。在主函数中,演示了如何使用 `SM4Utils` 类进行加密解密。注意,这里使用的是 Apache Commons Codec 库来进行 Base64 编解码。
阅读全文
相关推荐
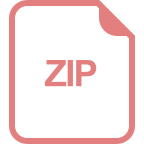
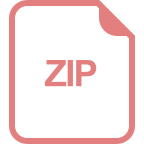
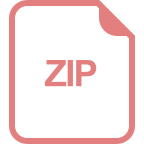
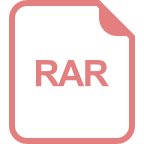
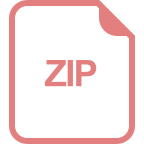
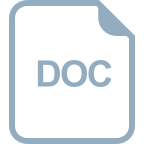








