使用psutil库实现这个功能
时间: 2023-07-20 09:11:04 浏览: 190
可以使用Python的`psutil`库来实现定时关闭程序的进程。`psutil`库是一个跨平台的进程和系统监控库,可以方便地获取和操作进程的信息。
具体步骤如下:
1. 使用`psutil`模块启动程序进程;
2. 使用`os`模块获取当前时间,计算出程序需要在多长时间后关闭;
3. 使用`time.sleep`函数阻塞程序,等待定时时间到达;
4. 使用`psutil`模块关闭程序进程。
以下是示例代码:
```python
import psutil
import os
import time
# 启动程序进程
process = psutil.Popen(['python', 'your_program.py'])
# 获取当前时间和定时时间
current_time = time.time()
shutdown_time = current_time + 60 * 10 # 10分钟后关闭程序
# 阻塞程序,等待定时时间到达
while time.time() < shutdown_time:
time.sleep(1)
# 关闭程序进程
process.terminate()
```
在上面的代码中,`your_program.py`是要运行的程序,`shutdown_time`是程序需要在多长时间后关闭,这里设置为10分钟。可以根据需要调整这个时间。
相关问题
python使用psutil库实现监控当前执行程序x.exe的内存占用峰值,并输出到x.txt中
以下是实现该功能的Python代码:
```python
import psutil
process_name = "x.exe"
peak_memory = 0
for proc in psutil.process_iter():
if proc.name() == process_name:
pid = proc.pid
process = psutil.Process(pid)
memory_usage = process.memory_info().rss
if memory_usage > peak_memory:
peak_memory = memory_usage
with open("x.txt", "w") as f:
f.write(str(peak_memory))
```
该代码使用psutil库中的process_iter()方法遍历所有正在运行的进程,找到名称为"x.exe"的进程并获取其进程ID(pid)。然后使用pid获取进程的内存使用情况,并将峰值内存占用记录在变量peak_memory中。最后,将峰值内存占用写入x.txt文件中。
python psutil 库
psutil是一个跨平台的Python库,用于检索有关运行中进程和系统利用率(CPU,内存,磁盘,网络,传感器)的信息。它可以帮助我们实现系统监控、进程管理、性能分析等功能。以下是psutil库的一些基本用法:
1.安装psutil库
```shell
pip install psutil
```
2.获取CPU信息
```python
import psutil
# 获取CPU逻辑数量
print("CPU逻辑数量:", psutil.cpu_count())
# 获取CPU物理核心
print("CPU物理核心:", psutil.cpu_count(logical=False))
# 获取CPU使用率,每秒刷新一次,累计10次
for i in range(10):
print("CPU使用率:", psutil.cpu_percent(interval=1, percpu=True))
```
3.获取内存信息
```python
import psutil
# 获取内存信息
mem = psutil.virtual_memory()
print("内存总量:", mem.total)
print("内存使用量:", mem.used)
print("内存空闲量:", mem.free)
print("内存使用率:", mem.percent)
```
4.获取磁盘信息
```python
import psutil
# 获取磁盘分区信息
partitions = psutil.disk_partitions()
for partition in partitions:
print("磁盘分区:", partition.device)
print("文件系统类型:", partition.fstype)
print("磁盘分区挂载点:", partition.mountpoint)
print("磁盘分区总容量:", psutil.disk_usage(partition.mountpoint).total)
print("磁盘分区使用容量:", psutil.disk_usage(partition.mountpoint).used)
print("磁盘分区空闲容量:", psutil.disk_usage(partition.mountpoint).free)
print("磁盘分区使用率:", psutil.disk_usage(partition.mountpoint).percent)
```
5.获取网络信息
```python
import psutil
# 获取网络接口信息
net_io = psutil.net_io_counters()
print("网络总字节数:", net_io.bytes_sent + net_io.bytes_recv)
print("网络总包数:", net_io.packets_sent + net_io.packets_recv)
# 获取网络连接信息
connections = psutil.net_connections()
for conn in connections:
print("本地地址:", conn.laddr)
print("远程地址:", conn.raddr)
print("状态:", conn.status)
```
阅读全文
相关推荐
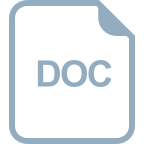
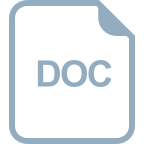
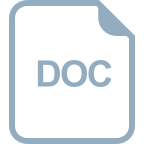
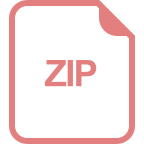
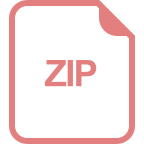
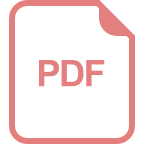
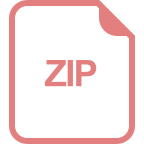
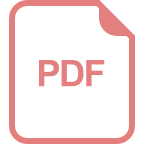
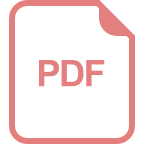
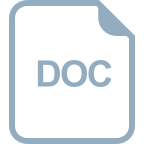






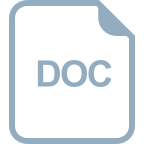