python 本地 实时图片rtmp推流
时间: 2023-10-11 22:08:52 浏览: 58
要实现本地实时图片 RTMP 推流,可以使用 Python 的 OpenCV 库进行实时图片捕获和处理,以及使用 FFmpeg 库进行 RTMP 推流。
以下是一个简单的 Python 代码示例,可以实现本地实时图片实时捕获并通过 RTMP 推流到指定的流媒体服务器:
```python
import cv2
import subprocess
import numpy as np
# 设置图片宽高和 FPS
width = 640
height = 360
fps = 30
# 设置视频编码格式
fourcc = cv2.VideoWriter_fourcc(*'X264')
# 设置推流地址和推流参数
rtmp_url = 'rtmp://example.com/live/stream'
rtmp_args = [
'-f', 'flv',
'-vcodec', 'libx264',
'-preset', 'veryfast',
'-tune', 'zerolatency',
'-b', '900k',
'-s', f'{width}x{height}',
'-r', str(fps),
rtmp_url
]
# 打开 FFmpeg 进程
process = subprocess.Popen(['ffmpeg', '-y', '-f', 'rawvideo', '-s', f'{width}x{height}', '-pix_fmt', 'bgr24', '-r', str(fps), '-i', '-', *rtmp_args], stdin=subprocess.PIPE)
while True:
# 生成一张随机图片
img = np.random.randint(0, 255, (height, width, 3), dtype=np.uint8)
# 对图片进行处理
# ...
# 将图片写入 FFmpeg 进程的 stdin
process.stdin.write(img.tobytes())
# 关闭 FFmpeg 进程
process.stdin.close()
process.wait()
```
需要注意的是,以上代码仅作为示例,实际使用时可能需要根据具体情况进行调整和优化。同时,需要安装 OpenCV 和 FFmpeg 库,并且确保推流地址和参数正确。
阅读全文
相关推荐




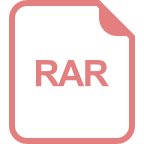
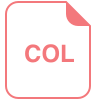
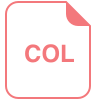







