Java WebSocket实现简易聊天室
时间: 2023-08-14 07:06:32 浏览: 69
Java WebSocket可以用于实现简易聊天室。下面是一个简单的示例代码:
1. 首先,创建一个WebSocketServer类,继承自javax.websocket.Endpoint,实现onOpen、onMessage、onClose和onError方法。
```java
import javax.websocket.*;
import javax.websocket.server.ServerEndpoint;
import java.io.IOException;
import java.util.concurrent.CopyOnWriteArraySet;
@ServerEndpoint("/chatRoom")
public class WebSocketServer {
//在线人数
private static int onlineCount = 0;
//存储每个客户端对应的WebSocketServer对象
private static CopyOnWriteArraySet<WebSocketServer> webSocketSet = new CopyOnWriteArraySet<>();
//与某个客户端的会话,需要通过它来给客户端发送数据
private Session session;
@OnOpen
public void onOpen(Session session) {
this.session = session;
//将WebSocketServer对象加入到webSocketSet中
webSocketSet.add(this);
//在线人数加1
addOnlineCount();
System.out.println("有新连接加入!当前在线人数为" + getOnlineCount());
}
@OnClose
public void onClose() {
//将WebSocketServer对象从webSocketSet中移除
webSocketSet.remove(this);
//在线人数减1
subOnlineCount();
System.out.println("有一连接关闭!当前在线人数为" + getOnlineCount());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("来自客户端的消息:" + message);
//群发消息
for (WebSocketServer webSocketServer : webSocketSet) {
try {
webSocketServer.sendMessage(message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
@OnError
public void onError(Session session, Throwable error) {
System.out.println("发生错误:" + error.getMessage());
error.printStackTrace();
}
public void sendMessage(String message) throws IOException {
this.session.getBasicRemote().sendText(message);
}
public static synchronized int getOnlineCount() {
return onlineCount;
}
public static synchronized void addOnlineCount() {
WebSocketServer.onlineCount++;
}
public static synchronized void subOnlineCount() {
WebSocketServer.onlineCount--;
}
}
```
2. 在web.xml中配置WebSocketServlet。
```xml
<servlet>
<servlet-name>WebSocketServlet</servlet-name>
<servlet-class>org.apache.tomcat.websocket.server.WsSci</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
```
3. 在jsp页面中添加WebSocket连接。
```html
var websocket = new WebSocket("ws://" + window.location.host + "/chatRoom");
websocket.onopen = function () {
console.log("WebSocket连接成功!");
};
websocket.onmessage = function (event) {
console.log("接收到服务器发送的消息:" + event.data);
};
websocket.onclose = function () {
console.log("WebSocket连接关闭!");
};
websocket.onerror = function () {
console.log("WebSocket连接发生错误!");
};
```
4. 发送消息。
```html
<button onclick="send()">发送</button>
<script>
function send() {
var message = document.getElementById("message").value;
websocket.send(message);
}
</script>
```
这样,一个简易聊天室就实现了。多个用户可以在同一页面中实时聊天。
相关推荐
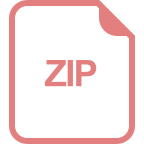
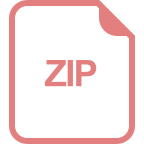
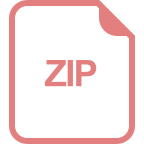
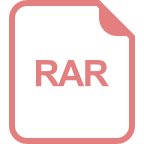
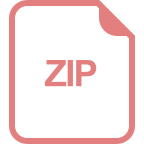
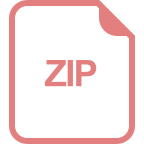
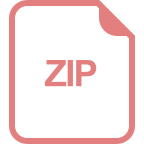
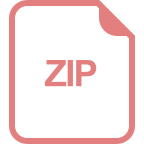
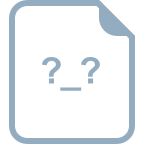
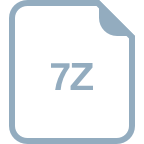