C语言实现元素1,2,3,4,5的进栈
时间: 2023-09-30 17:04:48 浏览: 89
以下是C语言实现元素1,2,3,4,5的进栈的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 5
int stack[MAX_SIZE];
int top = -1;
void push(int element) {
if (top == MAX_SIZE - 1) {
printf("Stack is full, cannot push anymore.\n");
return;
}
top++;
stack[top] = element;
printf("%d has been pushed to the stack.\n", element);
}
int main() {
push(1);
push(2);
push(3);
push(4);
push(5);
return 0;
}
```
该代码定义了一个固定大小为5的栈,使用`push`函数将元素依次进栈。在每次调用`push`函数时,会检查栈是否已满,如果已满则输出提示信息并返回。在`main`函数中依次调用`push`函数将元素1,2,3,4,5进栈。
相关问题
c语言实现元素1,2,3,4,5的进栈
以下是C语言实现元素1,2,3,4,5的进栈的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 5
int stack[MAX_SIZE];
int top = -1;
void push(int element) {
if (top == MAX_SIZE - 1) {
printf("Stack is full, cannot push anymore.\n");
return;
}
top++;
stack[top] = element;
printf("%d has been pushed to the stack.\n", element);
}
int main() {
push(1);
push(2);
push(3);
push(4);
push(5);
return 0;
}
```
该代码定义了一个固定大小为5的栈,使用`push`函数将元素依次进栈。在每次调用`push`函数时,会检查栈是否已满,如果已满则输出提示信息并返回。在`main`函数中依次调用`push`函数将元素1,2,3,4,5进栈。
c语言实现元素1,2,3,4,5的进栈,出栈次序为2,1,5,4,3
以下是使用 C 语言实现栈的代码,实现元素1,2,3,4,5的进栈,出栈次序为2,1,5,4,3:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 5
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int x) {
if (is_full(s)) {
printf("Stack is full.\n");
return;
}
s->top++;
s->data[s->top] = x;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty.\n");
return -1;
}
int x = s->data[s->top];
s->top--;
return x;
}
int main() {
Stack s;
init(&s);
// 进栈1,2,3,4,5
push(&s, 1);
push(&s, 2);
push(&s, 3);
push(&s, 4);
push(&s, 5);
// 出栈2,1,5,4,3
printf("%d ", pop(&s)); // 2
printf("%d ", pop(&s)); // 1
printf("%d ", pop(&s)); // 5
printf("%d ", pop(&s)); // 4
printf("%d ", pop(&s)); // 3
return 0;
}
```
阅读全文
相关推荐
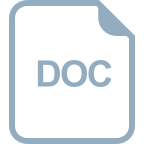
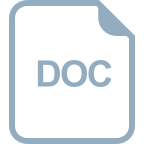
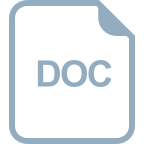













