vue2商品价格升降排序和删除
时间: 2023-11-17 22:08:20 浏览: 31
好的,针对你的问题,我可以给你提供一些指导。
1. 商品价格升降排序:
如果你使用的是 Vue 2.x,你可以使用 `v-for` 指令来循环渲染商品列表,并且在 `v-for` 指令中使用 `v-bind` 指令来绑定商品价格。例如:
```
<div v-for="product in products" :key="product.id">
<p>{{ product.name }}</p>
<p>价格:{{ product.price }}</p>
</div>
```
如果你想要实现价格排序,你可以在 Vue 组件的 `methods` 选项中定义一个排序的方法,并在模板中使用 `v-on:click` 指令来绑定这个方法。例如:
```
<template>
<div>
<button @click="sortAscending">价格从低到高</button>
<button @click="sortDescending">价格从高到低</button>
<div v-for="product in sortedProducts" :key="product.id">
<p>{{ product.name }}</p>
<p>价格:{{ product.price }}</p>
</div>
</div>
</template>
<script>
export default {
data() {
return {
products: [
{ id: 1, name: '商品 A', price: 100 },
{ id: 2, name: '商品 B', price: 200 },
{ id: 3, name: '商品 C', price: 300 }
]
}
},
methods: {
sortAscending() {
this.products.sort((a, b) => a.price - b.price)
},
sortDescending() {
this.products.sort((a, b) => b.price - a.price)
}
},
computed: {
sortedProducts() {
return this.products.slice()
}
}
}
</script>
```
在这个例子中,我们定义了两个排序方法 `sortAscending` 和 `sortDescending`,分别对应价格从低到高和价格从高到低排序。我们还定义了一个计算属性 `sortedProducts`,用来返回已经排序的商品列表。在模板中,我们使用 `v-for` 指令来循环渲染 `sortedProducts`,并绑定每个商品的 `id` 作为 `key`。
2. 商品删除:
如果你想要实现商品删除,你可以在模板中使用 `v-on:click` 指令来绑定一个删除方法。例如:
```
<template>
<div>
<div v-for="product in products" :key="product.id">
<p>{{ product.name }}</p>
<p>价格:{{ product.price }}</p>
<button @click="removeProduct(product)">删除</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
products: [
{ id: 1, name: '商品 A', price: 100 },
{ id: 2, name: '商品 B', price: 200 },
{ id: 3, name: '商品 C', price: 300 }
]
}
},
methods: {
removeProduct(product) {
const index = this.products.indexOf(product)
this.products.splice(index, 1)
}
}
}
</script>
```
在这个例子中,我们在模板中为每个商品渲染一个“删除”按钮,并绑定一个 `removeProduct` 方法来处理删除操作。在这个方法中,我们使用 `Array.prototype.indexOf()` 函数来找到要删除的商品在商品列表中的索引,然后使用 `Array.prototype.splice()` 函数来从商品列表中删除这个商品。
希望以上内容能够对你有所帮助!
相关推荐
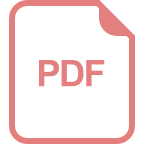














