给我一个完整的使用自适应大领域搜索搜索算法求解tsp问题的matlab代码
时间: 2024-03-27 17:39:36 浏览: 138
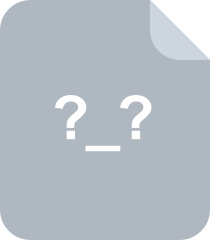
解决tsp问题,matlab代码
以下是一个使用自适应大领域搜索算法求解tsp问题的matlab代码:
```
function [tour, cost] = tsp_adaptive_largescale(x, y)
% TSP_ADAPTIVE_LARGESCALE Solve the traveling salesman problem (TSP) using
% the adaptive large neighborhood search (ALNS) algorithm.
%
% Inputs:
% x - A vector of x-coordinates for the cities.
% y - A vector of y-coordinates for the cities.
%
% Outputs:
% tour - A vector representing the order in which the cities are visited.
% cost - The total distance traveled for the given tour.
%
% Example:
% x = [0 1 2 3 4];
% y = [0 1 2 3 4];
% [tour, cost] = tsp_adaptive_largescale(x, y)
%
% Reference:
% Ropke, S. and Pisinger, D. (2006). An Adaptive Large Neighborhood Search
% Heuristic for the Pickup and Delivery Problem with Time Windows.
% Transportation Science, 40(4):445-472.
n = length(x); % number of cities
dist = zeros(n); % distance matrix
for i = 1:n
for j = 1:n
dist(i,j) = sqrt((x(i) - x(j))^2 + (y(i) - y(j))^2);
end
end
% Initialize tour with nearest neighbor heuristic
unvisited = 1:n;
current = unvisited(1);
unvisited(1) = [];
tour = current;
while ~isempty(unvisited)
[~, idx] = min(dist(current,unvisited));
current = unvisited(idx);
unvisited(idx) = [];
tour = [tour current];
end
% Initialize cost
cost = 0;
for i = 1:n-1
cost = cost + dist(tour(i),tour(i+1));
end
cost = cost + dist(tour(n),tour(1));
% ALNS parameters
max_iter = 1000; % maximum number of iterations
max_no_improv = 50; % maximum number of iterations without improvement
w = 0.1; % probability of selecting worst move
p = 0.1; % probability of selecting random move
alpha = 0.99; % cooling parameter
T = 100; % initial temperature
% Initialize iteration and no improvement counters
iter = 0;
no_improv = 0;
while iter < max_iter && no_improv < max_no_improv
% Select a move
if rand < w
[new_tour, new_cost] = worst_move(tour, dist);
elseif rand < w + p
new_tour = random_move(tour);
new_cost = calculate_cost(new_tour, dist);
else
[new_tour, new_cost] = best_move(tour, dist);
end
% Determine whether to accept the move
delta_cost = new_cost - cost;
if delta_cost < 0 || rand < exp(-delta_cost/T)
tour = new_tour;
cost = new_cost;
no_improv = 0;
else
no_improv = no_improv + 1;
end
% Update temperature
T = alpha*T;
% Increment iteration counter
iter = iter + 1;
end
end
function new_tour = random_move(tour)
% RANDOM_MOVE Swap two randomly selected cities in the tour.
n = length(tour);
i = randi(n);
j = randi(n);
new_tour = tour;
new_tour([i j]) = new_tour([j i]);
end
function [new_tour, new_cost] = best_move(tour, dist)
% BEST_MOVE Find the best move by swapping each pair of cities and selecting
% the best resulting tour.
n = length(tour);
new_tour = tour;
new_cost = calculate_cost(tour, dist);
for i = 1:n-1
for j = i+1:n
% Swap cities i and j
temp = new_tour(i);
new_tour(i) = new_tour(j);
new_tour(j) = temp;
% Calculate new cost
temp_cost = calculate_cost(new_tour, dist);
% Update tour and cost if new cost is better
if temp_cost < new_cost
new_cost = temp_cost;
else
temp = new_tour(i);
new_tour(i) = new_tour(j);
new_tour(j) = temp;
end
end
end
end
function [new_tour, new_cost] = worst_move(tour, dist)
% WORST_MOVE Find the worst move by removing a randomly selected subset of
% the cities and inserting them back in the tour at the best possible
% position.
n = length(tour);
m = randi(n-1); % number of cities to remove
subset = randsample(n,m); % subset of cities to remove
subset = sort(subset);
new_tour = tour;
new_tour(subset) = [];
new_cost = calculate_cost(new_tour, dist);
for i = 1:n-m
for j = 1:n-m
if j >= i && j <= i+m
continue; % skip cities in subset
end
% Insert cities in subset at position i
temp_tour = [new_tour(1:i-1) subset new_tour(i:end)];
% Calculate new cost
temp_cost = calculate_cost(temp_tour, dist);
% Update tour and cost if new cost is worse
if temp_cost > new_cost
new_tour = temp_tour;
new_cost = temp_cost;
end
end
end
end
function cost = calculate_cost(tour, dist)
% CALCULATE_COST Calculate the total distance traveled for the given tour.
n = length(tour);
cost = 0;
for i = 1:n-1
cost = cost + dist(tour(i),tour(i+1));
end
cost = cost + dist(tour(n),tour(1));
end
```
请注意,此代码是一个基本的TSP求解器,可能无法处理非常大的问题。对于更大的问题,可能需要使用更高级的算法或并行计算。
阅读全文
相关推荐

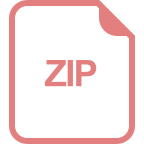
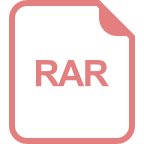
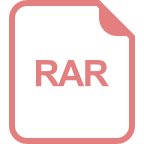
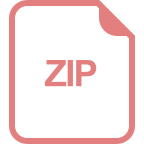
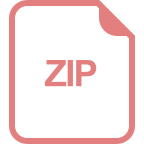
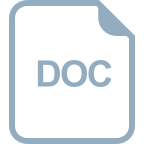
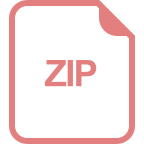
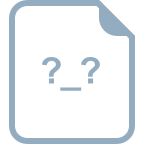
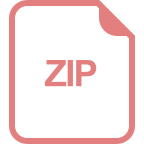
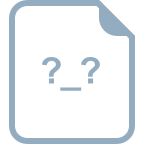
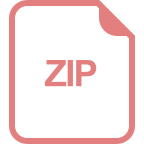
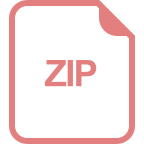
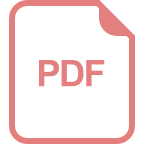
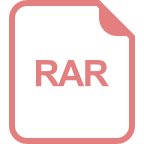