请使用C++字符串流结合常用STL容器完成以下功能: 统计这段文字的不重复的单词个数(即多次出现的单词只计为一个) 纯数字的单字不作为单词 注意本题要求:
时间: 2023-06-17 13:03:46 浏览: 43
以下是使用C++字符串流结合常用STL容器实现统计不重复单词个数的代码:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <unordered_set>
int main()
{
std::string text = "Hello world! This is a sample text for testing string stream and STL container usage. Hello world!";
std::istringstream iss(text);
std::unordered_set<std::string> word_set;
std::string word;
while (iss >> word)
{
// Check if the word contains only alphabets
bool is_alpha = true;
for (char c : word)
{
if (!isalpha(c))
{
is_alpha = false;
break;
}
}
// If the word contains only alphabets and is not already in the set, add it to the set
if (is_alpha && word_set.find(word) == word_set.end())
{
word_set.insert(word);
}
}
std::cout << "Number of unique words: " << word_set.size() << std::endl;
return 0;
}
```
该程序将输入的文本字符串存入字符串流中,然后使用unordered_set容器存储不重复的单词。在遍历字符串流中的单词时,首先检查该单词是否只包含字母,如果是则检查该单词是否已经存在于unordered_set中,不存在则将其添加到unordered_set中。最后输出unordered_set的大小即为不重复单词的个数。
相关推荐
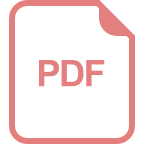
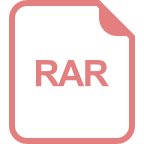
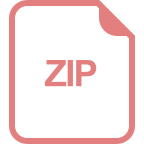














