请使用fork、exec、wait、exit函数实现
时间: 2023-09-18 22:08:35 浏览: 186
这个任务需要使用多个系统调用来实现,下面是一个简单的示例程序:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid;
int status;
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid == 0) {
// 子进程
printf("Child process is running\n");
execl("/bin/ls", "ls", "-l", NULL);
perror("exec");
exit(EXIT_FAILURE);
} else {
// 父进程
printf("Parent process is waiting for child process\n");
wait(&status);
printf("Child process exited with status %d\n", WEXITSTATUS(status));
exit(EXIT_SUCCESS);
}
}
```
这个程序会创建一个子进程,然后在子进程中执行 `ls -l` 命令,最后等待子进程结束并输出子进程返回的状态码。注意,`execl` 函数会用新的程序替换当前进程,所以在子进程中 `execl` 函数后面的代码不会被执行。如果 `execl` 函数执行成功,它不会返回,否则会返回 -1。
另外,需要注意的是,`wait` 函数会阻塞当前进程,直到它的子进程退出为止。如果在父进程中没有调用 `wait` 函数或者调用了但是子进程还没有退出,那么子进程就会变成僵尸进程。所以,在实际生产环境中,需要特别注意避免僵尸进程的产生。
阅读全文
相关推荐
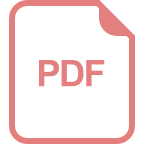
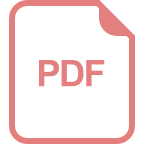
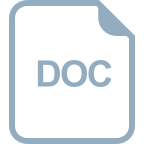
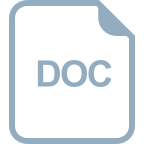
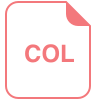

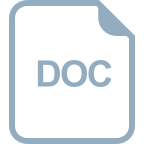
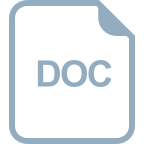
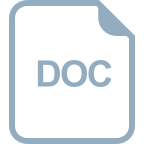







