假定算数表达式的值均为非负整数常数,不包含变量、小 数和字符常量。 试设计一个算术四则运算表达式求值的简单计算器。 基本要求: 1、 操作数均为非负整数常数,操作符仅为+、-、*、/、(、); 2、 编写 main 函数进行测试
时间: 2023-11-08 19:58:25 浏览: 66
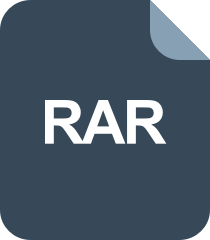
简单的四则运算的计算器
ChitGPT:
您好,我是ChitGPT,一个类ChatGPT的AI,很高兴能和您进行对话。关于您的问题,我可以为您提供以下代码,用于实现一个算术四则运算表达式求值的简单计算器:
```
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool is_operator(char op) {
return op == '+' || op == '-' || op == '*' || op == '/';
}
int priority(char op) {
if (op == '*' || op == '/')
return 2;
else if (op == '+' || op == '-')
return 1;
else
return 0;
}
int calc(int a, int b, char op) {
if (op == '+')
return a + b;
else if (op == '-')
return a - b;
else if (op == '*')
return a * b;
else
return a / b;
}
int evaluate_expression(string exp) {
stack<int> nums;
stack<char> ops;
int i = 0;
while (i < exp.length()) {
if (isdigit(exp[i])) {
int num = 0;
while (i < exp.length() && isdigit(exp[i])) {
num = num * 10 + (int)(exp[i] - '0');
i++;
}
nums.push(num);
}
else if (exp[i] == '(') {
ops.push(exp[i]);
i++;
}
else if (exp[i] == ')') {
while (!ops.empty() && ops.top() != '(') {
int b = nums.top();
nums.pop();
int a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
int res = calc(a, b, op);
nums.push(res);
}
ops.pop();
i++;
}
else if (is_operator(exp[i])) {
char op = exp[i];
while (!ops.empty() && priority(op) <= priority(ops.top())) {
int b = nums.top();
nums.pop();
int a = nums.top();
nums.pop();
char prev_op = ops.top();
ops.pop();
int res = calc(a, b, prev_op);
nums.push(res);
}
ops.push(op);
i++;
}
else {
i++;
}
}
while (!ops.empty()) {
int b = nums.top();
nums.pop();
int a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
int res = calc(a, b, op);
nums.push(res);
}
return nums.top();
}
int main() {
string exp = "3+4*5-(6+7)*8";
int res = evaluate_expression(exp);
cout << "Expression: " << exp << endl;
cout << "Result: " << res << endl;
return 0;
}
```
请注意,此代码不包含输入表达式,您需要自己在代码中指定要处理的表达式。另外,请确保您已在代码中包含了必要的头文件,并使用了正确的命名空间。如果您有任何问题,请随时与我联系。
阅读全文
相关推荐
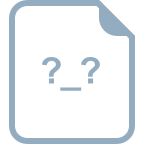
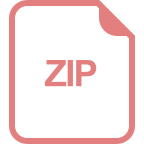

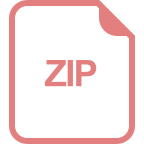
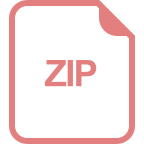
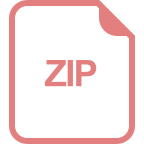
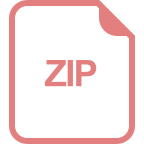
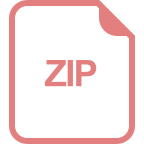
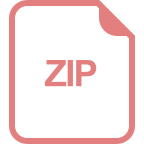
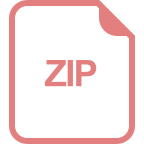
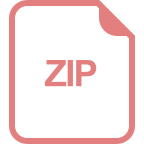
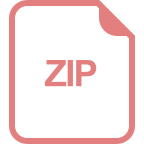
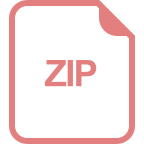
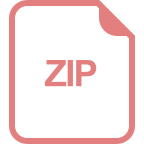