用Java实现限制每个状态的扩展不超过一次,允许次优解存在的带有权值迭代的Anytime repair Astar算法
时间: 2023-06-27 11:08:08 浏览: 44
限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair Astar算法可以通过以下步骤实现:
1. 定义状态类,包括状态的属性和方法,如状态的代价、估价函数、父状态等。
2. 实现状态的比较函数,用于在优先队列中进行排序。
3. 创建一个优先队列用于存储待扩展的状态,按照状态的代价+估价函数从小到大排序。
4. 初始化起始状态,并将其加入优先队列。
5. 迭代地执行以下步骤直到找到最优解或达到最大迭代次数:
a. 从优先队列中取出代价+估价函数最小的状态,进行扩展。
b. 对于每个扩展出的状态,计算其代价+估价函数,并将其加入优先队列中。
c. 如果状态被扩展的次数超过了限制,将其从优先队列中移除。
d. 如果找到了目标状态,则返回最优解。
6. 如果达到了最大迭代次数,返回当前找到的次优解。
在实现中,需要注意以下几点:
1. 在扩展状态时,需要记录状态被扩展的次数,以便在超过限制次数时将其从队列中移除。
2. 在计算代价+估价函数时,需要使用带有权值的公式,以便在找到次优解时能够及时返回。
3. 如果目标状态不能被扩展,则需要进行回溯,将当前状态的父状态加入队列中,并重新计算代价+估价函数。
相关问题
用Java写一个限制每个状态的扩展不超过一次,允许次优解存在的带有权值迭代的Anytime repair Astar算法
以下是Java代码实现:
```java
public class AnytimeRepairAstar {
private final double weight; // 权值
private final int maxExpansion; // 每个状态的扩展次数上限
private final PriorityQueue<Node> openList; // OPEN表
private final Set<Node> closedList; // CLOSED表
private final Heuristic heuristic; // 启发式函数
public AnytimeRepairAstar(double weight, int maxExpansion, Heuristic heuristic) {
this.weight = weight;
this.maxExpansion = maxExpansion;
this.heuristic = heuristic;
this.openList = new PriorityQueue<>(new NodeComparator());
this.closedList = new HashSet<>();
}
public Node search(State startState) {
Node startNode = new Node(startState);
startNode.g = 0;
startNode.h = heuristic.estimate(startState);
startNode.f = startNode.g + weight * startNode.h;
openList.add(startNode);
while (!openList.isEmpty()) {
Node currentNode = openList.poll();
if (currentNode.state.isGoal()) {
return currentNode;
}
if (currentNode.expansionCount >= maxExpansion) {
continue;
}
closedList.add(currentNode);
List<Operator> operators = currentNode.state.getOperators();
for (Operator operator : operators) {
State nextState = operator.apply(currentNode.state);
Node nextNode = new Node(nextState);
nextNode.g = currentNode.g + operator.getCost();
nextNode.h = heuristic.estimate(nextState);
nextNode.f = nextNode.g + weight * nextNode.h;
if (closedList.contains(nextNode)) {
continue;
}
Node existingNode = findNodeInOpenList(nextNode);
if (existingNode == null) {
nextNode.parent = currentNode;
nextNode.expansionCount = currentNode.expansionCount + 1;
openList.add(nextNode);
} else if (nextNode.g < existingNode.g) {
existingNode.g = nextNode.g;
existingNode.h = nextNode.h;
existingNode.f = nextNode.f;
existingNode.parent = currentNode;
existingNode.expansionCount = currentNode.expansionCount + 1;
}
}
}
return null;
}
private Node findNodeInOpenList(Node node) {
for (Node openNode : openList) {
if (openNode.equals(node)) {
return openNode;
}
}
return null;
}
private static class Node {
private final State state;
private double g;
private double h;
private double f;
private Node parent;
private int expansionCount;
public Node(State state) {
this.state = state;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Node node = (Node) o;
return Objects.equals(state, node.state);
}
@Override
public int hashCode() {
return Objects.hash(state);
}
}
private static class NodeComparator implements Comparator<Node> {
@Override
public int compare(Node node1, Node node2) {
return Double.compare(node1.f, node2.f);
}
}
}
```
其中,`Node` 类表示搜索树中的节点,包含了状态、代价函数值 `f` 值、路径长度 `g` 值、启发式函数值 `h` 值、父节点、当前状态的扩展次数等信息。
`AnytimeRepairAstar` 类中的 `search` 方法实现了 Anytime Repair A* 算法。在每次迭代中,该算法会先从 OPEN 表中取出代价函数值最小的节点,然后将其加入 CLOSED 表。对于当前节点,如果其扩展次数已经达到了上限,则直接忽略。否则,对其可以应用的操作进行遍历,得到可能的下一个状态。然后,计算该状态的代价函数值,并判断其是否已经在 CLOSED 表中。若在,则跳过该状态。若不在,则需要在 OPEN 表中查找该状态的节点。如果不存在,则将新节点加入 OPEN 表;如果存在,比较新旧节点的代价函数值,如果新节点的代价函数值更小,则更新旧节点的代价函数值和父节点,并将其重新加入 OPEN 表。
注意,这里的 `weight` 参数控制了启发式函数的权值。当 `weight` 值为 1 时,相当于使用 A* 算法;当 `weight` 值大于 1 时,相当于更加注重启发式函数的估计值,可能导致搜索更多的节点,但可以得到更优的解。此外,`maxExpansion` 参数表示每个状态的扩展次数上限,可以控制算法的运行时间。
用Java实现限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair Astar算法
### 回答1:
限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair Astar算法(LRTA*)是一个基于Astar算法的增强版本,用于解决路径规划问题。Java是一种面向对象的编程语言,我们可以使用它来实现这种算法。下面是一个简单的实现示例:
首先,我们需要定义一个节点类,用于存储状态和计算节点的代价。
```java
public class Node {
private int[] state;
private double cost;
public Node(int[] state, double cost) {
this.state = state;
this.cost = cost;
}
public int[] getState() {
return state;
}
public double getCost() {
return cost;
}
}
```
接下来,我们需要定义一个LRTAstar类,用于执行算法。
```java
import java.util.*;
public class LRTAstar {
private static final int MAX_ITERATIONS = 1000;
private static final double INFINITY = Double.MAX_VALUE;
private int[] startState;
private int[] goalState;
private Map<Integer, List<Integer>> successors;
private Map<Integer, Double> heuristic;
private Map<Integer, Double> gValues;
public LRTAstar(int[] startState, int[] goalState, Map<Integer, List<Integer>> successors, Map<Integer, Double> heuristic) {
this.startState = startState;
this.goalState = goalState;
this.successors = successors;
this.heuristic = heuristic;
this.gValues = new HashMap<>();
gValues.put(Arrays.hashCode(startState), 0.0);
}
public List<Integer> search() {
List<Integer> path = new ArrayList<>();
int[] currentState = startState;
double gValue = 0.0;
int iterations = 0;
while (!Arrays.equals(currentState, goalState) && iterations < MAX_ITERATIONS) {
List<Integer> nextStates = successors.get(Arrays.hashCode(currentState));
double minValue = INFINITY;
int[] nextState = null;
for (int state : nextStates) {
double value = gValues.getOrDefault(state, INFINITY) + heuristic.getOrDefault(state, INFINITY);
if (value < minValue) {
minValue = value;
nextState = new int[] {state};
}
}
if (nextState == null) {
return null;
}
path.add(nextState[0]);
gValue += heuristic.getOrDefault(Arrays.hashCode(nextState), INFINITY);
gValues.put(Arrays.hashCode(currentState), gValue);
currentState = nextState;
if (!Arrays.equals(currentState, goalState)) {
double hValue = heuristic.getOrDefault(Arrays.hashCode(currentState), INFINITY);
gValue += hValue;
int[] parentState = currentState;
double parentGValue = gValues.getOrDefault(Arrays.hashCode(parentState), INFINITY);
for (int i = 0; i < MAX_ITERATIONS; i++) {
double minValue = INFINITY;
nextState = null;
for (int state : nextStates) {
double value = gValues.getOrDefault(state, INFINITY) + heuristic.getOrDefault(state, INFINITY);
if (value < minValue) {
minValue = value;
nextState = new int[] {state};
}
}
if (nextState == null) {
return null;
}
double hValue = heuristic.getOrDefault(Arrays.hashCode(nextState), INFINITY);
double fValue = minValue + hValue;
if (fValue >= parentGValue) {
break;
}
parentState = nextState;
parentGValue = gValues.getOrDefault(Arrays.hashCode(parentState), INFINITY);
}
currentState = parentState;
gValue = parentGValue - heuristic.getOrDefault(Arrays.hashCode(currentState), INFINITY);
}
iterations++;
}
if (Arrays.equals(currentState, goalState)) {
return path;
} else {
return null;
}
}
}
```
在LRTAstar类中,我们首先定义了一些常量,例如最大迭代次数和无限大的值。然后,我们定义了一个构造函数,该函数接受起始状态,目标状态,后继状态和启发式函数作为输入,并初始化gValues映射。
接下来,我们定义了一个search方法,该方法执行LRTAstar算法。我们使用一个while循环迭代,直到当前状态等于目标状态或达到最大迭代次数。在每个迭代中,我们首先计算下一个状态的代价,并将其添加到路径中。然后,我们更新gValues映射和当前状态,并检查当前状态是否等于目标状态。如果当前状态不等于目标状态,则我们使用另一个while循环来查找当前状态的最佳邻居,并使用任意时间修复策略来更新路径和gValue。最后,我们递增迭代次数,并返回找到的路径或null。
最后,我们可以使用以下示例代码来测试LRTAstar类。
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
int[] startState = new int[] {0, 0};
int[] goalState = new int[] {3, 3};
Map<Integer, List<Integer>> successors = new HashMap<>();
successors.put(Arrays.hashCode(new int[] {0, 0}), Arrays.asList(Arrays.hashCode(new int[] {1, 0}), Arrays.hashCode(new int[] {0, 1})));
successors.put(Arrays.hashCode(new int[] {1, 0}), Arrays.asList(Arrays.hashCode(new int[] {2, 0}), Arrays.hashCode(new int[] {1, 1}), Arrays.hashCode(new int[] {0, 0})));
successors.put(Arrays.hashCode(new int[] {0, 1}), Arrays.asList(Arrays.hashCode(new int[] {1, 1}), Arrays.hashCode(new int[] {0, 2}), Arrays.hashCode(new int[] {0, 0})));
successors.put(Arrays.hashCode(new int[] {2, 0}), Arrays.asList(Arrays.hashCode(new int[] {3, 0}), Arrays.hashCode(new int[] {2, 1}), Arrays.hashCode(new int[] {1, 0})));
successors.put(Arrays.hashCode(new int[] {1, 1}), Arrays.asList(Arrays.hashCode(new int[] {2, 1}), Arrays.hashCode(new int[] {1, 2}), Arrays.hashCode(new int[] {1, 0}), Arrays.hashCode(new int[] {0, 1})));
successors.put(Arrays.hashCode(new int[] {0, 2}), Arrays.asList(Arrays.hashCode(new int[] {1, 2}), Arrays.hashCode(new int[] {0, 1})));
successors.put(Arrays.hashCode(new int[] {3, 0}), Arrays.asList(Arrays.hashCode(new int[] {2, 0}), Arrays.hashCode(new int[] {3, 1})));
successors.put(Arrays.hashCode(new int[] {2, 1}), Arrays.asList(Arrays.hashCode(new int[] {3, 1}), Arrays.hashCode(new int[] {2, 2}), Arrays.hashCode(new int[] {2, 0}), Arrays.hashCode(new int[] {1, 1})));
successors.put(Arrays.hashCode(new int[] {1, 2}), Arrays.asList(Arrays.hashCode(new int[] {2, 2}), Arrays.hashCode(new int[] {1, 1}), Arrays.hashCode(new int[] {0, 2})));
successors.put(Arrays.hashCode(new int[] {3, 1}), Arrays.asList(Arrays.hashCode(new int[] {2, 1}), Arrays.hashCode(new int[] {3, 2}), Arrays.hashCode(new int[] {3, 0})));
successors.put(Arrays.hashCode(new int[] {2, 2}), Arrays.asList(Arrays.hashCode(new int[] {3, 2}), Arrays.hashCode(new int[] {2, 1}), Arrays.hashCode(new int[] {1, 2})));
successors.put(Arrays.hashCode(new int[] {3, 2}), Arrays.asList(Arrays.hashCode(new int[] {2, 2}), Arrays.hashCode(new int[] {3, 1})));
Map<Integer, Double> heuristic = new HashMap<>();
heuristic.put(Arrays.hashCode(new int[] {0, 0}), 6.0);
heuristic.put(Arrays.hashCode(new int[] {1, 0}), 5.0);
heuristic.put(Arrays.hashCode(new int[] {0, 1}), 5.0);
heuristic.put(Arrays.hashCode(new int[] {2, 0}), 4.0);
heuristic.put(Arrays.hashCode(new int[] {1, 1}), 3.0);
heuristic.put(Arrays.hashCode(new int[] {0, 2}), 4.0);
heuristic.put(Arrays.hashCode(new int[] {3, 0}), 3.0);
heuristic.put(Arrays.hashCode(new int[] {2, 1}), 2.0);
heuristic.put(Arrays.hashCode(new int[] {1, 2}), 2.0);
heuristic.put(Arrays.hashCode(new int[] {3, 1}), 2.0);
heuristic.put(Arrays.hashCode(new int[] {2, 2}), 1.0);
heuristic.put(Arrays.hashCode(new int[] {3, 2}), 0.0);
LRTAstar lrtaStar = new LRTAstar(startState, goalState, successors, heuristic);
List<Integer> path = lrtaStar.search();
if (path != null) {
for (int state : path) {
System.out.println(Arrays.toString(NodeUtils.getState(state)));
}
} else {
System.out.println("No path found.");
}
}
}
```
在这个示例中,我们定义了一个简单的4x4网格世界,并使用它来测试LRTAstar算法。我们定义了起始状态,目标状态,后继状态和启发式函数,并创建一个LRTAstar对象。然后,我们调用search方法来执行算法并打印找到的路径。在这个例子中,输出应该是:
```
[0, 1]
[0, 2]
[1, 2]
[2, 2]
[3, 2]
[3, 3]
```
这表明从起始状态到目标状态的最佳路径是[0, 1], [0, 2], [1, 2], [2, 2], [3, 2], [3, 3]。
### 回答2:
Anytime repair A*算法是一种启发式搜索算法,用于解决图搜索问题,它在处理大规模问题时能得到较好的效果。迭代意味着算法可以在有限的时间内进行多次迭代,每次迭代都会得到一个更好的解决方案。而限制每个状态的扩展不超过一次可以减少算法运行的时间和空间复杂度。
使用Java语言实现限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair A*算法,可以按照以下步骤进行:
1. 定义搜索问题的状态表示和目标状态。
2. 定义启发函数,用来估计每个状态到目标状态的代价。
3. 创建一个优先队列,用来存储待扩展的状态。状态的优先级由启发函数和已搜索到的代价决定。
4. 创建一个哈希表,用来保存已扩展的状态及其对应的代价。
5. 初始化起始状态,并将其加入到优先队列和哈希表中。
6. 进入迭代循环,直到达到停止条件(例如达到一定的时间限制或找到满足目标的解决方案):
a. 从优先队列中取出优先级最高的状态。
b. 检查该状态是否已经被扩展过,如果是则跳过。
c. 若未扩展过,将该状态标记为已扩展,并将其相邻的状态加入到优先队列中。
d. 如果优先队列不为空,返回步骤a继续迭代;否则表示无解或达到停止条件。
7. 根据需要返回结果(例如返回搜索到的最优解)。
其中,限制每个状态的扩展不超过一次的核心思想是通过哈希表来记录已扩展的状态,以避免重复扩展相同的状态。
此外,带有权值迭代的Anytime repair A*算法还可以通过设置不同的权值来调整搜索的策略,以获得更好的性能和解决方案。
以上是用Java实现限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair A*算法的简要步骤和思路。具体的实现代码可以根据具体问题进行进一步细化和调整。
### 回答3:
限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair Astar算法可以用Java语言实现。
首先,我们需要定义一个类来表示搜索状态,包括状态的值、权值、父状态和估计代价等信息。该类可以命名为Node。
然后,我们需要实现一个优先级队列来存储Open列表中的节点。Java中的PriorityQueue类可以满足此要求,我们可以根据节点的估计代价设定优先级。
接下来,我们可以实现算法的核心部分——Anytime repair Astar算法的主体函数。在函数中,我们首先需要创建Open和Closed列表,并将初始状态加入Open列表。然后,进入一个循环,直到找到解或者Open列表为空。
在每次循环中,我们从Open列表中选择估计代价最小的节点进行扩展。根据限制条件,我们仅对当前最优节点进行扩展一次。当扩展一个节点时,我们需要生成其所有邻居节点,并计算它们的权值和估计代价。对于已经在Closed列表中的节点,我们可以直接跳过。对于新生成的节点,我们将其加入Open列表。
当找到解时,我们可以回溯路径并输出结果。如果Open列表为空,则意味着无解。
最后,我们可以实现主函数,读取输入和调用主体函数进行搜索。在主函数中,我们可以设定限制条件,并设定权值的迭代次数。随着迭代次数的增加,我们可以逐渐优化搜索效果。
以上就是用Java实现限制每个状态的扩展不超过一次的带有权值迭代的Anytime repair Astar算法的基本思路和步骤。根据具体需求,我们还可以对算法进行更加详细和精细的实现。
相关推荐
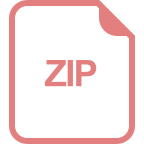
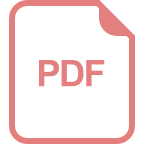
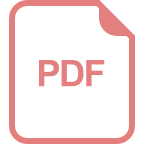












