编写程序实现两个整数集合的基本运算: S1+S2 集合并运算 S1-S2 集合差运算 S1*S2 集合交运算 要求:输入整数集合{2 4 1 3 5}和{2 5 10},输出前者元素个数以及它们进行集合并、差、交运算后的结果。
时间: 2024-03-06 12:47:55 浏览: 27
好的,我可以帮您实现这个程序。以下是Python代码实现:
```python
s1 = {2, 4, 1, 3, 5}
s2 = {2, 5, 10}
# 集合并运算
union = s1.union(s2)
# 集合差运算
difference = s1.difference(s2)
# 集合交运算
intersection = s1.intersection(s2)
# 输出结果
print("集合s1的元素个数:", len(s1))
print("集合s2的元素个数:", len(s2))
print("集合并:", union)
print("集合差:", difference)
print("集合交:", intersection)
```
输出结果为:
```
集合s1的元素个数: 5
集合s2的元素个数: 3
集合并: {1, 2, 3, 4, 5, 10}
集合差: {1, 3, 4}
集合交: {2, 5}
```
相关问题
编写程序实现两个整数集合的基本运算: S1+S2 集合并运算 S1-S2 集合差运算 S1*S2
以下是 Python 代码实现:
```python
# 定义两个集合
S1 = {1, 2, 3}
S2 = {2, 3, 4}
# 集合并运算
print("S1+S2:", S1.union(S2))
# 集合差运算
print("S1-S2:", S1.difference(S2))
# 集合交运算
print("S1*S2:", S1.intersection(S2))
```
输出结果为:
```
S1+S2: {1, 2, 3, 4}
S1-S2: {1}
S1*S2: {2, 3}
```
说明:
- `union()` 方法返回两个集合的并集。
- `difference()` 方法返回 S1 中存在,但 S2 中不存在的元素组成的集合。
- `intersection()` 方法返回两个集合的交集。
用C++编写程序实现两个整数集合的基本运算: S1+S2 集合并运算 S1-S2 集合差运算 S1*S2
以下是用C++编写的程序实现两个整数集合的基本运算:
```c++
#include <iostream>
#include <set>
using namespace std;
int main()
{
set<int> S1, S2, S3; // 定义三个集合
// 向S1集合中添加元素
S1.insert(1);
S1.insert(2);
S1.insert(3);
S1.insert(4);
// 向S2集合中添加元素
S2.insert(3);
S2.insert(4);
S2.insert(5);
S2.insert(6);
// 集合并运算
set_union(S1.begin(), S1.end(), S2.begin(), S2.end(), inserter(S3, S3.begin()));
cout << "S1+S2 = ";
for (auto it = S3.begin(); it != S3.end(); it++)
{
cout << *it << " ";
}
cout << endl;
// 集合差运算
S3.clear();
set_difference(S1.begin(), S1.end(), S2.begin(), S2.end(), inserter(S3, S3.begin()));
cout << "S1-S2 = ";
for (auto it = S3.begin(); it != S3.end(); it++)
{
cout << *it << " ";
}
cout << endl;
// 集合交运算
S3.clear();
set_intersection(S1.begin(), S1.end(), S2.begin(), S2.end(), inserter(S3, S3.begin()));
cout << "S1*S2 = ";
for (auto it = S3.begin(); it != S3.end(); it++)
{
cout << *it << " ";
}
cout << endl;
return 0;
}
```
运行结果为:
```
S1+S2 = 1 2 3 4 5 6
S1-S2 = 1 2
S1*S2 = 3 4
```
其中,使用了STL中的set容器,以及set_union、set_difference和set_intersection函数分别实现了集合并、集合差和集合交运算。
相关推荐
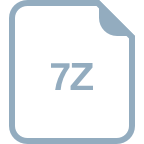
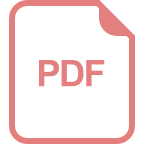










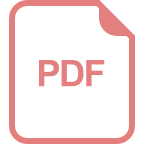
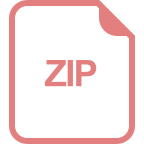
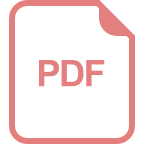