Python如何在子线程中使用qmessage
时间: 2023-03-14 09:09:48 浏览: 71
在Python中使用QMessage在子线程中需要使用QtCore.QObject.moveToThread()方法将QMessage实例移动到新的线程中,然后使用QtCore.QMetaObject.invokeMethod() 方法调用QMessage实例的方法。
相关问题
QMessageBox无法在线程中使用
您好!对于在线程中使用 QMessageBox,可以通过以下方法解决:
1. 在子线程中发送信号,然后在主线程中使用 QMessageBox。
```python
from PyQt5.QtCore import QThread, pyqtSignal
from PyQt5.QtWidgets import QMessageBox
class WorkerThread(QThread):
finished = pyqtSignal()
def run(self):
# 在这里执行耗时操作
self.finished.emit()
class MainWindow(QtWidgets.QMainWindow):
def __init__(self):
super().__init__()
self.thread = WorkerThread()
self.thread.finished.connect(self.show_message_box)
def show_message_box(self):
QMessageBox.information(self, "提示", "耗时操作已完成!")
def start_work(self):
self.thread.start()
```
2. 使用 QMetaObject.invokeMethod() 在主线程中调用 QMessageBox。
```python
from PyQt5.QtCore import QObject, QThread, pyqtSignal, QMetaObject
class WorkerThread(QThread):
finished = pyqtSignal()
def run(self):
# 在这里执行耗时操作
QMetaObject.invokeMethod(main_window, "show_message_box")
class MainWindow(QtWidgets.QMainWindow):
def __init__(self):
super().__init__()
def show_message_box(self):
QMessageBox.information(self, "提示", "耗时操作已完成!")
def start_work(self):
self.thread = WorkerThread()
self.thread.finished.connect(self.show_message_box)
self.thread.start()
```
这些方法都是将显示 QMessageBox 的操作放在主线程中执行,以避免在线程中直接使用 QMessageBox 导致的问题。希望对您有所帮助!
在子线程threading中 弹窗串口卡死
在子线程中弹窗会导致界面卡死的问题,这是因为 PyQt5 是单线程的 GUI 库,所有的 GUI 操作必须在主线程中执行。如果在子线程中执行 GUI 操作,会导致界面卡死。
解决这个问题的方法是,将弹窗操作放在主线程中执行,然后在弹窗中启动一个子线程来执行耗时的操作。这样,弹窗就不会被卡死了。
以下是一个示例代码:
```python
import threading
from PyQt5.QtCore import pyqtSignal, QObject
from PyQt5.QtWidgets import QApplication, QMessageBox
class Worker(QObject):
finished = pyqtSignal()
def __init__(self):
super().__init__()
def long_time_operation(self):
# 执行耗时的操作
self.finished.emit()
def show_message_box():
msg_box = QMessageBox()
msg_box.setText("确定要执行操作吗?")
msg_box.setStandardButtons(QMessageBox.Ok | QMessageBox.Cancel)
ret = msg_box.exec_()
if ret == QMessageBox.Ok:
# 在子线程中执行耗时的操作
worker = Worker()
worker_thread = threading.Thread(target=worker.long_time_operation)
worker_thread.start()
worker.finished.connect(worker_thread.quit)
worker.finished.connect(worker.deleteLater)
worker_thread.finished.connect(worker_thread.deleteLater)
worker_thread.start()
if __name__ == '__main__':
app = QApplication([])
show_message_box()
app.exec_()
```
在这个示例代码中,我们定义了一个 `Worker` 类来执行耗时的操作。在 `show_message_box` 函数中,我们先弹出一个消息框询问用户是否要执行操作,然后在用户点击确定后,在主线程中创建一个 `Worker` 对象,并将其放在一个子线程中执行。我们通过信号和槽机制来保证子线程执行完后可以安全退出。这样,就可以避免弹窗被卡死的问题。
相关推荐
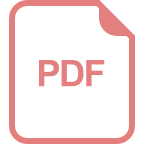
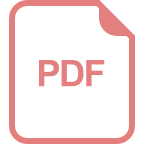
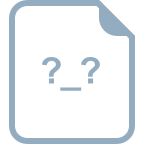













