调用mediapipe opencv手势识别,根据对应手势动态动作 给出代码
时间: 2024-01-01 08:03:29 浏览: 108
好的,下面是一个简单的示例代码,演示如何使用 Mediapipe 和 OpenCV 进行手势识别:
```python
import cv2
import mediapipe as mp
# 初始化 Mediapipe 手势识别模型
mp_hands = mp.solutions.hands
hands = mp_hands.Hands(static_image_mode=False, max_num_hands=2, min_detection_confidence=0.5, min_tracking_confidence=0.5)
# 初始化 OpenCV 视频捕获
cap = cv2.VideoCapture(0)
while True:
# 读取视频帧
ret, frame = cap.read()
# 将帧转换为 RGB 格式
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 使用 Mediapipe 进行手势识别
results = hands.process(frame)
# 绘制手势识别结果
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
# 在图像上绘制手部关键点
for id, lm in enumerate(hand_landmarks.landmark):
h, w, c = frame.shape
cx, cy = int(lm.x * w), int(lm.y * h)
cv2.circle(frame, (cx, cy), 5, (255, 0, 255), cv2.FILLED)
# 判断手势动作
# TODO: 根据手势动作执行相应的操作
# ...
# 将帧转换回 BGR 格式
frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR)
# 显示结果
cv2.imshow('Hand Gesture Detection', frame)
# 等待按下 ESC 键退出
if cv2.waitKey(1) == 27:
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
在上面的代码中,我们首先初始化了 Mediapipe 手势识别模型和 OpenCV 视频捕获。然后在循环中读取视频帧,使用 Mediapipe 进行手势识别,并在图像上绘制手势识别结果。最后根据手势动作执行相应的操作。你需要根据实际情况修改 TODO 部分的代码。
阅读全文
相关推荐
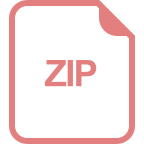
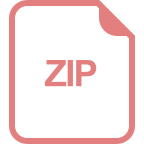
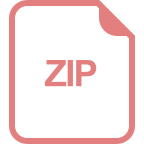
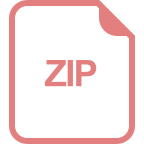
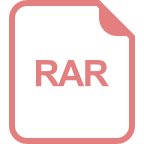
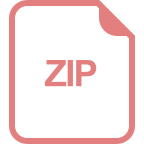
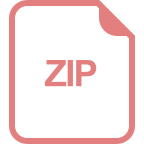
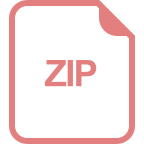
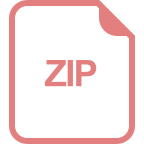
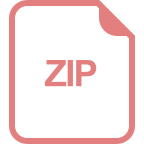
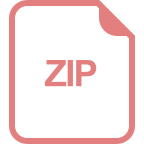
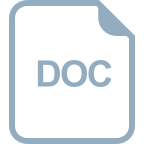
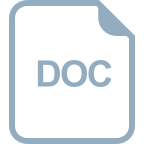
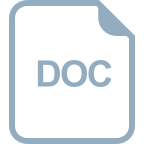
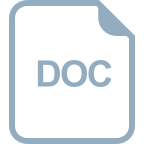
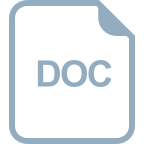

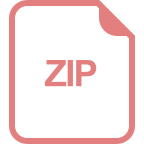