用pygame写个带有开始和结束的贪吃蛇小游戏
时间: 2024-02-25 19:59:41 浏览: 173
好的,下面是使用pygame写的带有开始和结束的贪吃蛇小游戏的示例代码:
```python
import pygame
import random
import sys
# 初始化pygame
pygame.init()
# 设置游戏窗口大小和标题
size = (640, 480)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("贪吃蛇")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 定义贪吃蛇的初始位置和大小
snake = [(320, 240), (310, 240), (300, 240)]
snake_size = 10
# 定义食物的初始位置
food = (random.randint(0, 63) * 10, random.randint(0, 47) * 10)
# 定义贪吃蛇的移动方向
direction = "RIGHT"
# 定义计分板的初始得分
score = 0
# 加载游戏需要用到的字体
font = pygame.font.SysFont("arial", 20)
# 加载游戏需要用到的音效
eat_sound = pygame.mixer.Sound("eat.wav")
gameover_sound = pygame.mixer.Sound("gameover.wav")
# 加载游戏需要用到的图片
bg_img = pygame.image.load("bg.png")
snake_img = pygame.image.load("snake.png")
food_img = pygame.image.load("food.png")
# 开始游戏
def start_game():
global snake, food, direction, score
snake = [(320, 240), (310, 240), (300, 240)]
food = (random.randint(0, 63) * 10, random.randint(0, 47) * 10)
direction = "RIGHT"
score = 0
# 游戏结束
def game_over():
global snake, food, direction, score
snake = []
food = ()
direction = ""
score_text = font.render("得分:" + str(score), True, WHITE)
screen.blit(score_text, (280, 200))
gameover_text = font.render("游戏结束!", True, WHITE)
screen.blit(gameover_text, (270, 230))
pygame.display.update()
pygame.time.delay(2000)
start_game()
# 进入游戏主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
start_game()
# 更新贪吃蛇的位置
head = snake[0]
if direction == "RIGHT":
new_head = (head[0] + snake_size, head[1])
elif direction == "LEFT":
new_head = (head[0] - snake_size, head[1])
elif direction == "UP":
new_head = (head[0], head[1] - snake_size)
elif direction == "DOWN":
new_head = (head[0], head[1] + snake_size)
snake.insert(0, new_head)
snake.pop()
# 判断是否吃到了食物
if new_head == food:
eat_sound.play()
snake.append(snake[-1])
food = (random.randint(0, 63) * 10, random.randint(0, 47) * 10)
score += 10
# 判断是否撞墙或撞到自己
if new_head[0] < 0 or new_head[0] > 630 or new_head[1] < 0 or new_head[1] > 470 or new_head in snake[1:]:
gameover_sound.play()
game_over()
# 绘制游戏界面
screen.blit(bg_img, (0, 0))
screen.blit(food_img, food)
for pos in snake:
screen.blit(snake_img, pos)
score_text = font.render("得分:" + str(score), True, WHITE)
screen.blit(score_text, (10, 10))
pygame.display.update()
# 处理键盘事件
keys = pygame.key.get_pressed()
if keys[pygame.K_RIGHT] and direction != "LEFT":
direction = "RIGHT"
elif keys[pygame.K_LEFT] and direction != "RIGHT":
direction = "LEFT"
elif keys[pygame.K_UP] and direction != "DOWN":
direction = "UP"
elif keys[pygame.K_DOWN] and direction != "UP":
direction = "DOWN"
```
在运行这段代码之前,你需要先准备好以下文件:
- `bg.png`:游戏背景图片
- `snake.png`:贪吃蛇图片
- `food.png`:食物图片
- `eat.wav`:吃到食物的音效
- `gameover.wav`:游戏结束的音效
这些文件需要和代码文件放在同一个目录下。你也可以根据需要修改这些文件的名称和路径。
阅读全文
相关推荐
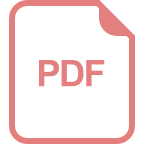
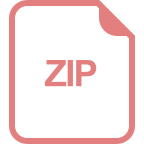
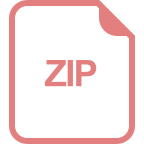
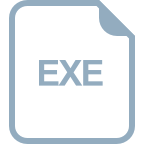
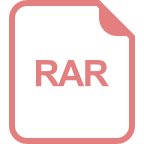
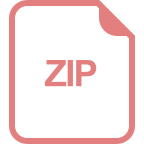
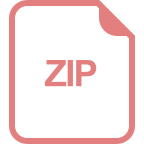
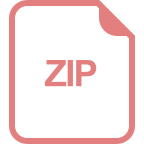