java批量导入,文字和图片实现同时批量导入
时间: 2024-05-06 21:19:03 浏览: 86
Java 批量导入文字和图片可以使用 Apache POI 和 Apache Commons IO 这两个开源库来实现。
首先需要导入这两个库的依赖:
```xml
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.0.1</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.0.1</version>
</dependency>
<!-- Apache Commons IO -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
```
接着,可以使用 POI 提供的 API 来读取 Excel 文件中的数据,并将图片保存到本地。下面是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.apache.poi.util.IOUtils;
public class ExcelImportDemo {
public static void main(String[] args) throws IOException {
// 读取 Excel 文件
Workbook workbook = WorkbookFactory.create(new File("data.xlsx"));
Sheet sheet = workbook.getSheetAt(0);
// 遍历每一行数据
for (Row row : sheet) {
// 获取文字数据
Cell textCell = row.getCell(0);
String text = textCell.getStringCellValue();
// 获取图片数据
Cell imageCell = row.getCell(1);
byte[] imageData = imageCell.getPictureData().getData();
// 将图片保存到本地文件
FileOutputStream outputStream = new FileOutputStream("image.png");
IOUtils.write(imageData, outputStream);
outputStream.close();
}
// 关闭 Excel 文件
workbook.close();
}
}
```
这个示例代码假设 Excel 文件的第一列为文字数据,第二列为图片数据。遍历每一行数据,将文字数据和图片数据分别取出来,然后将图片保存到本地文件中。你可以根据自己的需求修改代码,例如将图片直接插入到 Word 文档中等。
阅读全文
相关推荐
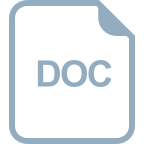
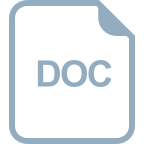
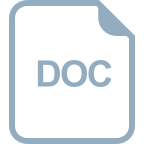
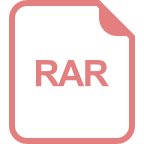
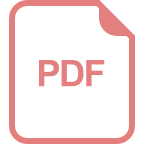
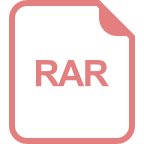
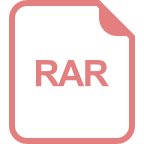
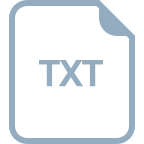
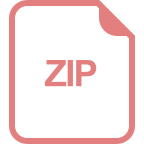
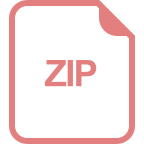
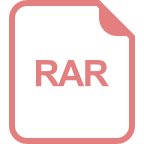
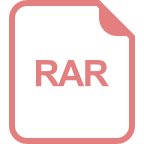
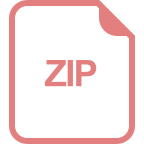
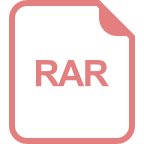
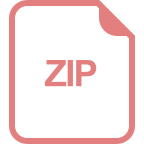
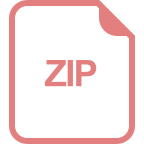
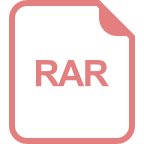
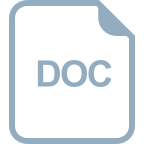
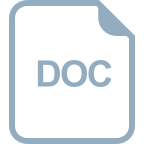