Build ensemble using the trained model by comtinued-value fusions(max and mean)
时间: 2024-03-18 12:42:40 浏览: 84
Sure, I can help you with that! To build an ensemble using the trained model by continued-value fusions (max and mean), you can follow these steps:
1. Load your trained models into memory.
2. Prepare your test data.
3. For each test example, feed it into each of the loaded models and obtain the output scores.
4. For each test example, calculate the maximum and mean scores across all the loaded models.
5. Use the maximum or mean score as the final prediction for each test example.
Here's some sample Python code to help get you started:
```python
import numpy as np
# Load your trained models into memory
model1 = load_model('model1.h5')
model2 = load_model('model2.h5')
model3 = load_model('model3.h5')
# Prepare your test data
test_data = ...
# Define a function to obtain the output scores for each model
def get_scores(model, test_data):
return model.predict(test_data)
# Obtain the output scores for each test example and each model
scores1 = get_scores(model1, test_data)
scores2 = get_scores(model2, test_data)
scores3 = get_scores(model3, test_data)
# Calculate the maximum and mean scores across all models for each test example
max_scores = np.maximum.reduce([scores1, scores2, scores3])
mean_scores = np.mean([scores1, scores2, scores3], axis=0)
# Use the maximum or mean score as the final prediction for each test example
final_predictions = np.argmax(max_scores, axis=1)
```
Note that this is just a simple example and you may need to modify it to suit your specific needs.
阅读全文
相关推荐
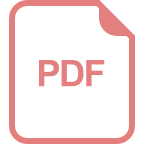
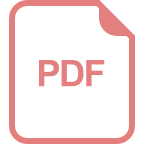

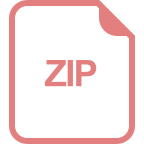
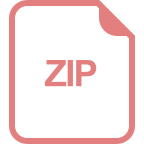
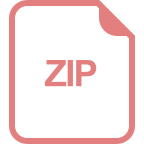
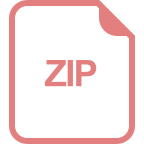
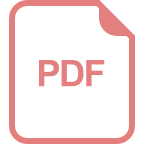
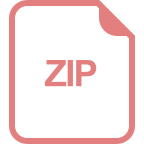
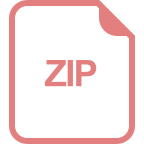
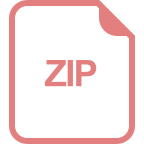
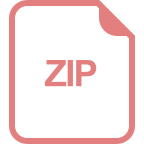
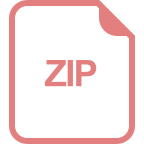
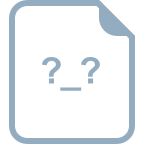
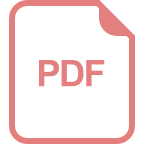
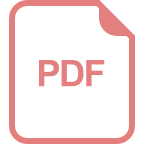
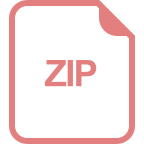