编写一个函数void SelectionSort(int score[], int n),用选择排序法实现从低到高排序
时间: 2024-02-24 10:55:53 浏览: 38
好的,下面是函数的实现:
```C++
void SelectionSort(int score[], int n)
{
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (score[j] < score[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
int temp = score[i];
score[i] = score[minIndex];
score[minIndex] = temp;
}
}
}
```
该函数的参数是一个整型数组 score 和数组的长度 n。它使用选择排序算法将数组从低到高排序。具体实现方法是,遍历数组中的每个元素,找到最小元素,并将其与该元素交换。这样,每次遍历后,数组中的最小元素都会被放到正确的位置上,直到整个数组都被排序完成。
相关问题
编写一个c程序,用函数 void sort(int *a,int n)对 a 开始的 n 个数从大到小排序。
好的,下面是实现该功能的 C 代码:
```
#include <stdio.h>
void sort(int *a, int n);
int main() {
int a[] = {4, 2, 1, 5, 3};
int n = 5;
sort(a, n);
printf("排序后的结果为:\n");
for (int i = 0; i < n; i++) {
printf("%d ", a[i]);
}
printf("\n");
return 0;
}
void sort(int *a, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (a[i] < a[j]) {
int temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
}
}
```
在 sort 函数中,我们使用了冒泡排序的思想,即从数组的第一个元素开始依次比较相邻的两个元素,如果前一个元素比后一个元素小,则交换它们的位置,直到整个数组都排好序。在主函数中,我们定义了一个整型数组 a 和数组的长度 n,然后调用 sort 函数对数组 a 进行排序,最后输出排序后的结果。
使用函数实现选择或冒泡排序:定义选择排序函数void selectsort(int a[],int n)
好的,以下是使用选择排序算法实现的 C++ 代码:
```
void selectsort(int a[], int n) {
int i, j, min_idx;
for (i = 0; i < n - 1; i++) {
min_idx = i;
for (j = i + 1; j < n; j++) {
if (a[j] < a[min_idx]) {
min_idx = j;
}
}
// Swap the minimum element with the first element
int temp = a[min_idx];
a[min_idx] = a[i];
a[i] = temp;
}
}
```
这个函数接收一个整数数组 `a` 和数组长度 `n` 作为参数,将数组按升序排序。算法的核心是在每一轮循环中找到当前未排序部分中的最小元素,然后与未排序部分的第一个元素交换位置。
你也可以使用类似的方式编写冒泡排序函数,只需要将算法实现替换为冒泡排序即可。
相关推荐
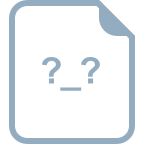












