Using Python to write genetic algorithm to calculate the maximum value of function f (x)=0.4+sinc (4x)+1.1sinc (4x+2)+0.8sinc (X-2)+0.7sinc (6x-4) between -2 and 2
时间: 2024-03-27 16:36:33 浏览: 15
Sure, I can help you with that! Here's one way you could implement a genetic algorithm to find the maximum value of the given function:
1. Define the fitness function: In this case, our fitness function will simply be the value of the function f(x) for a given value of x.
2. Generate an initial population: Create a set of random values of x within the range [-2, 2]. These values will be used as the starting point for the genetic algorithm.
3. Evaluate fitness: For each member of the population, calculate the fitness by evaluating the fitness function (i.e. calculate the value of f(x) for the given value of x).
4. Selection: Select the fittest individuals from the population to be the parents of the next generation. One simple way to do this is to use tournament selection: randomly select a few individuals from the population and choose the fittest one as a parent. Repeat this process until you have selected enough parents to create the next generation.
5. Crossover: Create new individuals for the next generation by combining the genes (i.e. values of x) of the selected parents. One common way to do this is to use single-point crossover: choose a random point in the genes and swap the genes from that point onwards between the two parents.
6. Mutation: Introduce random mutations into the genes of the new individuals to increase genetic diversity. One simple way to do this is to randomly select a gene and replace it with a new random value within the range [-2, 2].
7. Repeat: Repeat steps 3-6 until a stopping criteria is met (e.g. a maximum number of generations is reached, the fitness of the best individual reaches a certain threshold, etc.).
8. Output: Once the genetic algorithm has completed, output the best individual (i.e. the one with the highest fitness) and its corresponding value of x.
Here's some sample Python code to implement the genetic algorithm:
```python
import random
import math
# Define the fitness function
def fitness(x):
return 0.4 + math.sin(4*x)/4 + 1.1*math.sin(4*x+2)/4.4 + \
0.8*math.sin(x-2)/2.4 + 0.7*math.sin(6*x-4)/2.8
# Generate an initial population
POPULATION_SIZE = 100
population = [random.uniform(-2, 2) for _ in range(POPULATION_SIZE)]
# Genetic algorithm parameters
NUM_GENERATIONS = 1000
TOURNAMENT_SIZE = 5
MUTATION_RATE = 0.1
# Main loop
for generation in range(NUM_GENERATIONS):
# Evaluate fitness
fitness_scores = [fitness(x) for x in population]
# Selection
parents = []
for _ in range(POPULATION_SIZE):
tournament = random.sample(range(POPULATION_SIZE), TOURNAMENT_SIZE)
winner = max(tournament, key=lambda i: fitness_scores[i])
parents.append(population[winner])
# Crossover
new_population = []
for i in range(0, POPULATION_SIZE, 2):
parent1 = parents[i]
parent2 = parents[i+1]
crossover_point = random.randint(0, POPULATION_SIZE-1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
new_population.extend([child1, child2])
# Mutation
for i in range(POPULATION_SIZE):
if random.random() < MUTATION_RATE:
gene_index = random.randint(0, POPULATION_SIZE-1)
new_population[i][gene_index] = random.uniform(-2, 2)
# Replace old population with new population
population = new_population
# Output best individual
best_fitness = max(fitness_scores)
best_index = fitness_scores.index(best_fitness)
best_individual = population[best_index]
print("Best individual found:", best_individual)
print("Fitness:", best_fitness)
```
This code should give you an idea of how to implement a genetic algorithm to solve this problem. Note that the genetic algorithm is not guaranteed to find the global maximum of the function, as it may get stuck in a local optimum. Therefore, it's a good idea to run the algorithm multiple times with different random seeds to increase the chance of finding the global maximum.
相关推荐
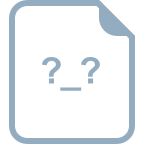
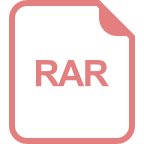














