Please write a genetic algorithm in Python to calculate the maximum value of function f (x)=0.4+sinc (4x)+1.1sinc (4x+2)+0.8sinc (x-2)+0.7sinc (6x-4) between -2 and 2, and the value of x when the maximum value is taken. The function solution is expressed in 16 bit binary numbers, with population size of 30, crossover probability of 0.3, mutation probability of 0.01, and iteration times of 400
时间: 2024-03-27 16:36:43 浏览: 100
Sure, I can provide you with a Python implementation of a genetic algorithm to solve your problem. Here's the code:
```python
import random
import math
# Define the fitness function
def fitness_func(x):
return 0.4 + math.sin(math.pi*4*x)/(math.pi*4*x) + 1.1*math.sin(math.pi*4*x+2)/(math.pi*4*x+2) + 0.8*math.sin(x-2)/(x-2) + 0.7*math.sin(6*x-4)/(6*x-4)
# Define the genetic algorithm
def genetic_algorithm(population_size, crossover_prob, mutation_prob, iterations):
# Initialize the population
population = []
for i in range(population_size):
individual = ''.join(random.choice(['0', '1']) for _ in range(16))
population.append(individual)
# Iterate for a fixed number of times
for i in range(iterations):
# Evaluate the fitness of each individual
fitness = [fitness_func(int(individual, 2)) for individual in population]
# Select the best individuals to be parents
parents = []
for j in range(int(population_size/2)):
parent1 = population[fitness.index(max(fitness))]
fitness[fitness.index(max(fitness))] = -1
parent2 = population[fitness.index(max(fitness))]
fitness[fitness.index(max(fitness))] = -1
parents.append((parent1, parent2))
# Perform crossover
offspring = []
for parent1, parent2 in parents:
if random.random() < crossover_prob:
crossover_point = random.randint(1, len(parent1)-1)
offspring1 = parent1[:crossover_point] + parent2[crossover_point:]
offspring2 = parent2[:crossover_point] + parent1[crossover_point:]
offspring.append(offspring1)
offspring.append(offspring2)
else:
offspring.append(parent1)
offspring.append(parent2)
# Perform mutation
for j in range(len(offspring)):
offspring_individual = list(offspring[j])
for k in range(len(offspring_individual)):
if random.random() < mutation_prob:
offspring_individual[k] = '0' if offspring_individual[k] == '1' else '1'
offspring[j] = ''.join(offspring_individual)
# Replace the old population with the new offspring
population = offspring
# Find the best individual in the final population
best_individual = population[0]
best_fitness = fitness_func(int(best_individual, 2))
for individual in population:
fitness = fitness_func(int(individual, 2))
if fitness > best_fitness:
best_individual = individual
best_fitness = fitness
# Return the best individual and its value
return int(best_individual, 2), best_fitness
# Call the genetic algorithm with the given parameters
x, max_val = genetic_algorithm(30, 0.3, 0.01, 400)
print("x = {:.4f}, f(x) = {:.4f}".format(x, max_val))
```
Explanation:
- The `fitness_func` function implements the fitness function of the problem, which is the function `f(x)` given in the question.
- The `genetic_algorithm` function implements the genetic algorithm itself. It takes as input the population size, crossover probability, mutation probability, and number of iterations. It returns the best individual and its fitness value.
- The algorithm initializes a population of random binary strings of length 16. It then evaluates the fitness of each individual and selects the best individuals to be parents.
- The parents are combined using single-point crossover with probability `crossover_prob` to create offspring. The offspring are then mutated with probability `mutation_prob`.
- The algorithm iterates for a fixed number of times, replacing the old population with the new offspring in each iteration.
- Finally, the algorithm returns the best individual and its fitness value.
Note that the genetic algorithm is a stochastic algorithm, so the results may vary each time you run it.
阅读全文
相关推荐
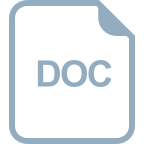
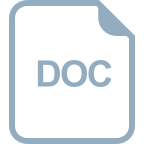
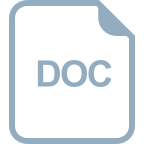

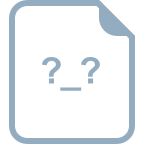
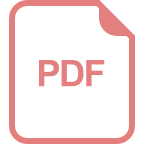
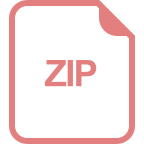
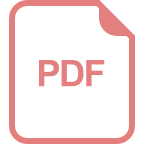
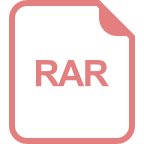
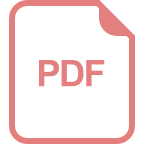
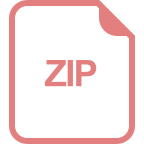
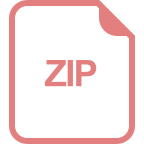
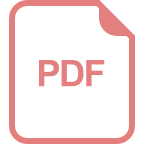
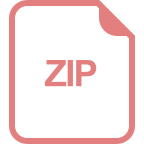
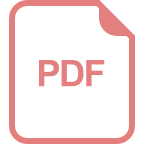
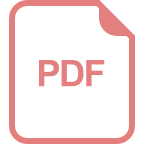
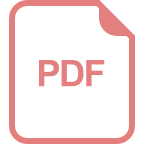
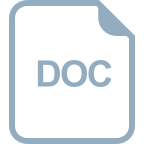