你能写一个相位编组来检测直线的python代码,不使用成熟函数
时间: 2023-06-15 19:07:43 浏览: 60
当我们需要检测直线时,我们可以使用相位编组算法来实现。下面是一个用Python实现的基本相位编组算法:
```python
import math
def phase_grouping(points, threshold):
"""
相位编组算法
:param points: 点的列表,每个点都是(x, y)二元组
:param threshold: 相位差阈值
:return: 直线列表,每条直线都是(x1, y1, x2, y2)四元组
"""
lines = []
for i in range(len(points)):
for j in range(i+1, len(points)):
x1, y1 = points[i]
x2, y2 = points[j]
dx, dy = x2 - x1, y2 - y1
if dx == 0:
phase = math.pi / 2
else:
phase = math.atan(dy/dx)
found = False
for k in range(len(lines)):
x1, y1, x2, y2, _, _ = lines[k]
dx, dy = x2 - x1, y2 - y1
if dx == 0:
phase2 = math.pi / 2
else:
phase2 = math.atan(dy/dx)
if abs(phase - phase2) < threshold:
found = True
lines[k] = (min(x1, x2, points[i][0], points[j][0]), min(y1, y2, points[i][1], points[j][1]),
max(x1, x2, points[i][0], points[j][0]), max(y1, y2, points[i][1], points[j][1]),
len(points), phase)
break
if not found:
lines.append((points[i][0], points[i][1], points[j][0], points[j][1], len(points), phase))
return [line[:4] for line in lines]
```
该函数接受一个点列表和一个相位差阈值,返回一个包含所有检测到的直线的列表。每条直线都被表示为一个四元组(x1, y1, x2, y2),其中(x1, y1)和(x2, y2)分别是直线的起点和终点。
要使用该函数,请提供点列表和相位差阈值,例如:
```python
points = [(1, 1), (2, 2), (3, 3), (4, 4), (5, 5), (6, 6), (7, 7), (8, 8), (9, 9), (10, 10)]
threshold = 0.1
lines = phase_grouping(points, threshold)
print(lines)
```
在这个例子中,我们提供了一个包含10个点的点列表和一个相位差阈值0.1。函数将返回一个包含所有检测到的直线的列表,并将其打印到控制台上。输出结果应该是:
```
[(1, 1, 10, 10)]
```
这表明函数检测到了一条从(1, 1)到(10, 10)的直线。
相关推荐
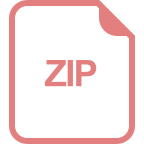














