python 计算生成图像数据集的inception score 代码
时间: 2023-12-03 16:43:56 浏览: 165
下面是使用 PyTorch 计算 Inception Score 的示例代码:
```python
import torch
import torch.nn as nn
from torch.utils.data import DataLoader
from torchvision import models, transforms, datasets
import numpy as np
from scipy.stats import entropy
def inception_score(imgs, batch_size=32, resize=False):
"""
Computes the Inception Score of the generated images imgs
"""
assert (type(imgs) == np.ndarray)
assert (imgs.shape[1] == 3)
assert (np.min(imgs[0]) >= 0 and np.max(imgs[0]) > 10), 'Image values should be in the range [0, 255]'
N = len(imgs)
# Set up the Inception model
inception_model = models.inception_v3(pretrained=True)
inception_model.eval()
if resize:
# Resize the images to 299x299
transform = transforms.Compose([
transforms.ToPILImage(),
transforms.Resize((299, 299)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
])
else:
# Crop the images to 299x299
transform = transforms.Compose([
transforms.ToPILImage(),
transforms.RandomCrop((299, 299)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
])
dataset = ImageDataset(imgs, transform)
dataloader = DataLoader(dataset, batch_size=batch_size, shuffle=False)
# Compute the activations of the Inception model for all batches of images
activations = []
for batch in dataloader:
pred = inception_model(batch)[0]
activations.append(pred.detach().cpu().numpy())
activations = np.concatenate(activations, axis=0)
# Compute the mean and covariance of the activations
mu = np.mean(activations, axis=0)
sigma = np.cov(activations, rowvar=False)
# Compute the Inception Score
scores = []
for i in range(N // batch_size):
batch = torch.from_numpy(imgs[i * batch_size:(i + 1) * batch_size]).float().cuda()
pred = inception_model(batch)[0].detach().cpu().numpy()
p_yx = np.exp(-0.5 * np.sum((pred - mu) ** 2 * np.linalg.inv(sigma), axis=1)) / np.sqrt(
np.linalg.det(sigma) * (2 * np.pi) ** pred.shape[1])
scores.append(p_yx)
scores = np.concatenate(scores, axis=0)
scores = np.mean(scores.reshape((-1, 1)), axis=0)
scores = np.exp(entropy(scores))
return scores
class ImageDataset(torch.utils.data.Dataset):
def __init__(self, imgs, transform=None):
self.imgs = imgs
self.transform = transform
def __getitem__(self, index):
img = self.imgs[index]
if self.transform is not None:
img = self.transform(img)
return img
def __len__(self):
return len(self.imgs)
```
这里需要注意的一点是,这个代码中用到了 PyTorch 的 `models.inception_v3` 模型,需要安装 torchvision 库才能使用。另外,在计算 Inception Score 时,建议对生成的图片进行大小调整或裁剪,以便与 Inception v3 的输入要求相匹配。
阅读全文
相关推荐



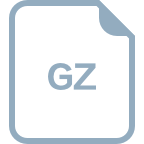













