请设计实现自己的字符串类(不要直接用系统 的string)自行设计补充 类中列出的方法的返回值,参数等自行设计实现。
时间: 2023-05-28 07:05:35 浏览: 70
以下是一个简单的字符串类的实现,包含了常见的基本方法:
```cpp
class MyString {
private:
char* data; // 存储字符串的字符数组
int length; // 字符串长度
public:
// 构造函数
MyString();
MyString(const char* str);
MyString(const MyString& other);
// 析构函数
~MyString();
// 赋值运算符重载
MyString& operator=(const char* str);
MyString& operator=(const MyString& other);
// 重载下标运算符
char& operator[](int index);
const char& operator[](int index) const;
// 获取字符串长度
int getLength() const;
// 判断字符串是否为空
bool isEmpty() const;
// 获取子串
MyString substring(int startIndex, int length) const;
// 查找子串在字符串中的位置
int indexOf(const char* str) const;
int indexOf(const MyString& str) const;
// 字符串拼接
MyString operator+(const char* str) const;
MyString operator+(const MyString& other) const;
// 字符串比较
bool operator==(const char* str) const;
bool operator==(const MyString& other) const;
bool operator!=(const char* str) const;
bool operator!=(const MyString& other) const;
bool operator>(const char* str) const;
bool operator>(const MyString& other) const;
bool operator<(const char* str) const;
bool operator<(const MyString& other) const;
bool operator>=(const char* str) const;
bool operator>=(const MyString& other) const;
bool operator<=(const char* str) const;
bool operator<=(const MyString& other) const;
// 插入子串
void insert(int index, const char* str);
void insert(int index, const MyString& str);
// 删除子串
void remove(int index, int length);
// 替换子串
void replace(const char* oldStr, const char* newStr);
void replace(const MyString& oldStr, const MyString& newStr);
// 转换大小写
void toLowerCase();
void toUpperCase();
// 转换为C风格字符串
const char* toCStr() const;
// 输出字符串
friend std::ostream& operator<<(std::ostream& os, const MyString& str);
};
```
其中,需要注意的一些细节包括:
- 构造函数应该初始化数据成员,并为字符串分配内存空间。
- 在构造函数和赋值运算符重载函数中,需要考虑字符串长度的变化和内存空间的重新分配。
- 在析构函数中,需要释放动态分配的内存空间。
- 重载下标运算符时,需要考虑越界情况。
- 获取子串时,需要考虑边界情况。
- 查找子串时,可以使用C++标准库中的`strstr`函数。
- 字符串拼接时,需要考虑内存空间的重新分配。
- 字符串比较时,可以使用C++标准库中的`strcmp`函数。
- 插入和删除子串时,需要考虑字符串长度的变化和内存空间的重新分配。
- 替换子串时,可以使用C++标准库中的`std::regex_replace`函数。
- 转换大小写时,可以使用C++标准库中的`std::tolower`和`std::toupper`函数。
- 转换为C风格字符串时,需要在字符串末尾添加一个空字符`\0`。
- 输出字符串时,可以使用C++标准库中的输出流。
相关推荐
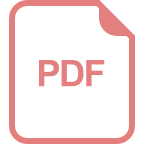
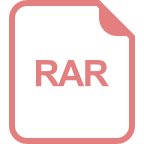
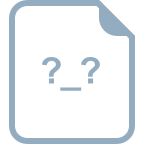








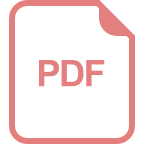
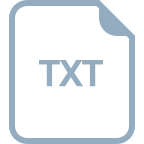
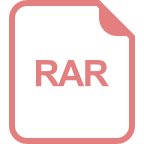
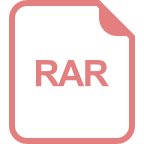
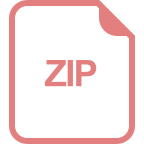
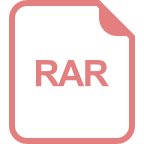