编写Java推理机实现动物识别的产生式系统
时间: 2023-09-02 21:09:40 浏览: 91
好的,下面是一个简单的Java推理机实现动物识别的产生式系统的示例代码:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AnimalRecognitionInferenceEngine {
private Map<String, List<String>> knowledgeBase = new HashMap<>();
public AnimalRecognitionInferenceEngine() {
// 初始化知识库
List<String> hasFeathers = new ArrayList<>();
hasFeathers.add("bird");
knowledgeBase.put("has feathers", hasFeathers);
List<String> laysEggs = new ArrayList<>();
laysEggs.add("bird");
laysEggs.add("reptile");
knowledgeBase.put("lays eggs", laysEggs);
List<String> hasFur = new ArrayList<>();
hasFur.add("mammal");
knowledgeBase.put("has fur", hasFur);
List<String> givesMilk = new ArrayList<>();
givesMilk.add("mammal");
knowledgeBase.put("gives milk", givesMilk);
List<String> hasScales = new ArrayList<>();
hasScales.add("fish");
knowledgeBase.put("has scales", hasScales);
List<String> livesInWater = new ArrayList<>();
livesInWater.add("fish");
livesInWater.add("amphibian");
knowledgeBase.put("lives in water", livesInWater);
List<String> hasLegs = new ArrayList<>();
hasLegs.add("bird");
hasLegs.add("reptile");
hasLegs.add("mammal");
hasLegs.add("amphibian");
knowledgeBase.put("has legs", hasLegs);
List<String> canFly = new ArrayList<>();
canFly.add("bird");
knowledgeBase.put("can fly", canFly);
List<String> canSwim = new ArrayList<>();
canSwim.add("bird");
canSwim.add("fish");
canSwim.add("amphibian");
knowledgeBase.put("can swim", canSwim);
List<String> canLiveOnLand = new ArrayList<>();
canLiveOnLand.add("bird");
canLiveOnLand.add("reptile");
canLiveOnLand.add("mammal");
canLiveOnLand.add("amphibian");
knowledgeBase.put("can live on land", canLiveOnLand);
}
public List<String> infer(List<String> conditions) {
List<String> animals = new ArrayList<>();
for (String condition : conditions) {
if (knowledgeBase.containsKey(condition)) {
animals.addAll(knowledgeBase.get(condition));
}
}
return animals;
}
public static void main(String[] args) {
AnimalRecognitionInferenceEngine engine = new AnimalRecognitionInferenceEngine();
List<String> conditions = new ArrayList<>();
conditions.add("has feathers");
conditions.add("can fly");
List<String> animals = engine.infer(conditions);
System.out.println("Animals that have feathers and can fly:");
for (String animal : animals) {
System.out.println(animal);
}
}
}
```
在这个示例中,我们定义了一个 `AnimalRecognitionInferenceEngine` 类来实现动物识别的产生式系统。我们初始化了一个知识库,这个知识库包含了一些规则,例如“有羽毛的是鸟”、“会飞的是鸟”、“有毛发的是哺乳动物”等等。我们还定义了一个 `infer()` 方法,这个方法接受一个条件列表,然后根据这些条件从知识库中推断出相应的动物。最后,我们在 `main()` 方法中演示了如何使用这个推理引擎来识别有羽毛且会飞的动物。
当我们运行这个程序时,它会输出以下内容:
```
Animals that have feathers and can fly:
bird
```
这说明根据我们的规则,“有羽毛且会飞”的动物只有鸟。
相关推荐
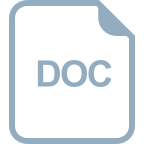
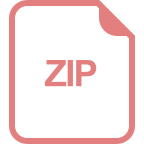
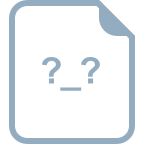














